使用 LinkedList或ArrayList 集合,实现编写一个模拟 KTV 点歌系统的程序,实现歌曲的添加、置顶、前移、后移、删除等操作。
时间: 2024-12-17 11:18:48 浏览: 7
在KTV点歌系统中,我们可以使用LinkedList和ArrayList这两种数据结构来实现歌曲列表的操作。这里我会简单地讲解如何设计这个系统,并提供每个功能的基本实现:
1. ArrayList:
ArrayList适合频繁的随机访问,比如快速查找某个歌曲的位置。可以将歌曲对象作为元素存储,然后使用get(), set()方法来进行操作。
```java
import java.util.*;
public class SongList {
private ArrayList<Song> songs;
public SongList() {
songs = new ArrayList<>();
}
// 添加歌曲
public void addSong(Song song) {
songs.add(song);
}
// 置顶歌曲
public void toTheTop(int index) {
if (index < 0 || index >= songs.size()) throw new IndexOutOfBoundsException();
Collections.swap(songs, 0, index);
}
// 前移歌曲
public void moveSong(int fromIndex, int toIndex) {
if (fromIndex == toIndex) return;
if (fromIndex < 0 || fromIndex >= songs.size() || toIndex < 0 || toIndex >= songs.size()) throw new IndexOutOfBoundsException();
Collections.move(songs, fromIndex, toIndex);
}
// 后移歌曲
public void moveSongToEnd(int index) {
if (index < 0 || index >= songs.size()) throw new IndexOutOfBoundsException();
songs.add(songs.remove(index));
}
// 删除歌曲
public void deleteSong(int index) {
if (index < 0 || index >= songs.size()) throw new IndexOutOfBoundsException();
songs.remove(index);
}
}
class Song { // 假设Song是一个简单的类,包含歌曲名和其他属性}
```
2. LinkedList:
LinkedList更适合频繁的插入和删除操作,因为它是通过节点链接的方式存储数据。对于上述操作,我们可以直接通过索引来操作链表节点。
```java
import java.util.LinkedList;
public class SongListLinkedList {
private LinkedList<Song> songs;
public SongListLinkedList() {
songs = new LinkedList<>();
}
// ... (类似上面的其他方法,只需修改set, get为LinkedList相应的方法)
public void addSong(Song song) {
songs.addLast(song);
}
// ... (链表的toTheTop, moveSong, moveSongToEnd, deleteSong都有现成方法,如removeFirst, removeAt等)
}
```
阅读全文
相关推荐
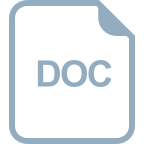
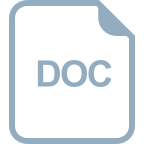
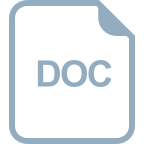



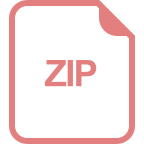
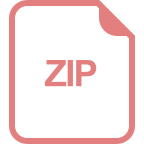
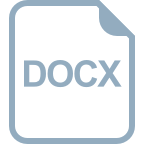
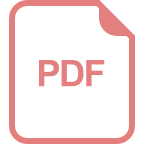
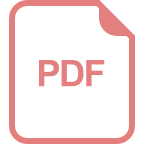
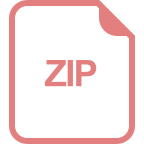
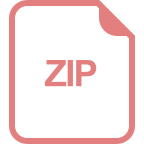
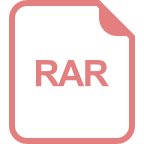
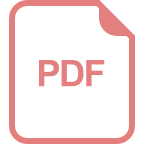
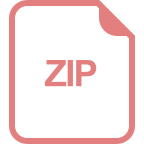
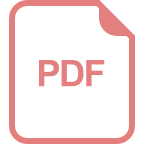
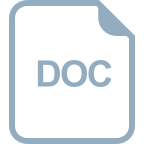