试实现函数matrix tr_convert(vector<int>ve),将上三角矩阵的压缩存储形式ve转化为原矩阵,结果作为返回值。
时间: 2024-10-14 17:04:47 浏览: 43
要实现这个函数 `matrix tr_convert(vector<int> ve)`,我们需要首先理解上三角矩阵的压缩存储表示(也称为“行主序”或“列主序”)。在这种表示法中,数组 `ve` 中的元素按照从上到下、从左到右的顺序存储非零元素,对于每个元素,我们可以通过索引计算其对应的矩阵中的行和列。
例如,如果原矩阵是:
```
1 2 0
3 4 5
0 6 7
```
对应的压缩存储 `ve` 可能是 `[1, 2, 3, 4, 5, 6, 7]`。
下面是实现该函数的一个C++示例:
```cpp
#include <vector>
// 定义一个二维矩阵模板
template <typename T>
class Matrix {
public:
int rows, cols;
std::vector<std::pair<int, T>> elements;
// 构造函数
Matrix(int r, int c) : rows(r), cols(c) {}
// 将压缩存储转换为矩阵
Matrix tr_convert(const std::vector<int>& ve) {
elements.clear();
for (size_t i = 0; i < ve.size(); ++i) {
if (i >= rows || ve[i] == 0) break;
elements.push_back({i, static_cast<T>(ve[i])});
}
return *this;
}
// 打印矩阵,用于测试
void print() const {
for (int row = 0; row < rows; ++row) {
for (const auto& elem : elements) {
if (elem.first <= row) {
std::cout << elem.second << ' ';
} else {
break;
}
}
std::cout << '\n';
}
}
};
// 示例用法
int main() {
std::vector<int> ve = {1, 2, 3, 4, 5, 6, 7};
Matrix<int> m(3, 3);
m.tr_convert(ve).print(); // 输出上三角矩阵
return 0;
}
```
在这个实现中,`Matrix` 类模板包含一个 `elements` 存储非零元素及其对应的行和列索引。`tr_convert` 函数接收一个 `vector` 并根据压缩存储重新填充 `elements` 后返回整个矩阵。`print` 方法用于打印矩阵进行验证。
阅读全文
相关推荐












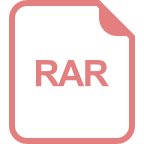



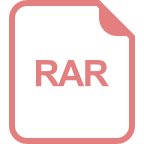
