以顺序表为存储结构实现以下基本操作: (1) 在第1个元素前插入一个新元素。 (2) 查找值为x的某个元素。若成功,给出x在表中的位置;不成功给出提示 信息。 (3)删除第1个元素,若成功,给出提示信息并显示被删元素的值;不成功给出失败的提示信息。用C++写出完整程序
时间: 2024-09-21 19:07:57 浏览: 30
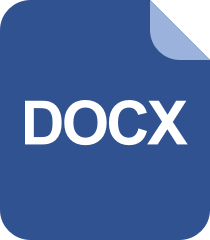
头歌数据结构顺序表的基本操作1-6关

为了以顺序表作为存储结构,在C++中实现上述基本操作,首先我们需要定义一个顺序表的节点结构体和包含相应操作的类。这里是一个简单的示例:
```cpp
#include <iostream>
using namespace std;
// 定义顺序表节点结构
struct Node {
int data;
Node* next;
};
class SequentialList {
private:
Node* head; // 链表头指针
int size; // 链表长度
public:
SequentialList() : head(nullptr), size(0) {}
// 插入元素到第一个位置
void insertAtStart(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = head;
head = newNode;
size++;
cout << "元素 " << value << " 已成功插入到第一位.\n";
}
// 查找元素并返回其索引
int findElement(int x) {
int index = -1;
Node* current = head;
for (int i = 0; current != nullptr && i < size; ++i, current = current->next) {
if (current->data == x) {
index = i;
break;
}
}
if (index != -1)
cout << "元素 " << x << " 所在位置: " << index + 1 << "\n";
else
cout << "未找到元素 " << x << ".\n";
return index;
}
// 删除第一个元素并输出其值
void deleteFirstElement() {
if (head == nullptr) {
cout << "链表为空,无法删除第一个元素.\n";
return;
}
int deletedValue = head->data;
Node* temp = head;
head = head->next;
delete temp;
size--;
cout << "已成功删除第一个元素,其值为: " << deletedValue << ".\n";
}
// 显示列表内容
void display() {
Node* current = head;
while (current != nullptr) {
cout << current->data << " -> ";
current = current->next;
}
cout << "NULL\n";
}
};
int main() {
SequentialList list;
// 测试操作
list.insertAtStart(5);
list.findElement(7);
list.insertAtStart(3);
list.display();
list.deleteFirstElement();
list.findElement(3);
return 0;
}
```
这个例子展示了如何创建一个顺序表并实现所需的操作。你可以将此代码复制到一个C++文件中运行它,观察各种操作的结果。
阅读全文
相关推荐
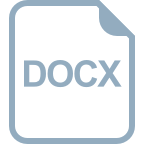
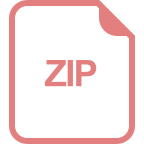






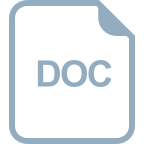
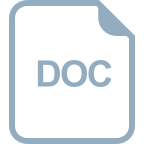







