黏菌算法Python代码
时间: 2024-09-24 18:26:27 浏览: 54
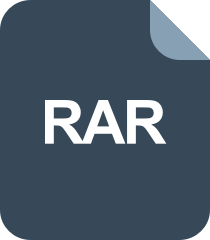
python编写细菌觅食优化算法(python代码)

黏菌( slime mold)是一种自然界的分布式计算模型,其行为受环境影响,通常用于模拟优化搜索问题,如最短路径、图像分割等。虽然没有直接的“黏菌算法”库像机器学习中的神经网络那样存在于Python标准库,但我们可以通过实现类似的行为逻辑来模拟。
以下是一个简单的Python代码示例,展示如何用列表或网格来模拟黏菌算法(也称为蚂蚁系统或土狼算法):
```python
import numpy as np
class Bacteria:
def __init__(self, position):
self.position = position
self.influence = 0.1
def update_bacteria(bacteria_list, grid_size):
# 更新每个细菌的位置
for bacteria in bacteria_list:
# 随机移动加上当前位置影响力
new_position = (bacteria.position[0] + np.random.choice([-1, 0, 1]),
bacteria.position[1] + np.random.choice([-1, 0, 1]))
if 0 <= new_position[0] < grid_size and 0 <= new_position[1] < grid_size:
bacteria.position = new_position
else:
bacteria.position = (grid_size - 1, grid_size - 1) # 边界处理
def apply_influence(grid, bacteria_list):
# 更新邻域细胞的值,考虑所有细菌的影响
for bacteria in bacteria_list:
for i, j in [(i, j) for i in range(-1, 2) for j in range(-1, 2) if (i != 0 or j != 0)]:
neighbor = (bacteria.position[0] + i, bacteria.position[1] + j)
if 0 <= neighbor[0] < len(grid) and 0 <= neighbor[1] < len(grid[0]):
grid[neighbor] += bacteria.influence
# 示例
grid = np.zeros((10, 10)) # 初始化网格
bacteria_list = [Bacteria(np.array([5, 5]))] # 添加初始细菌
for _ in range(100): # 迭代多次模拟过程
update_bacteria(bacteria_list, 10)
apply_influence(grid, bacteria_list)
print("Grid after simulation:", grid)
```
这个例子仅作为基本的模拟,实际应用可能需要更复杂的规则和迭代次数。请注意,这不是真正的黏菌算法,而是一个简化版本的模拟,主要用于教学目的。
阅读全文
相关推荐
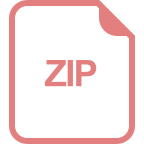
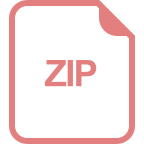

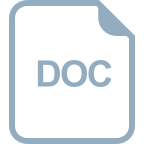





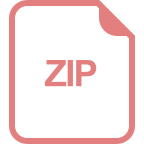
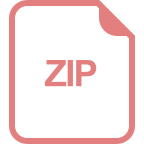
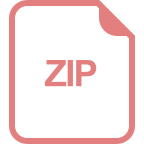
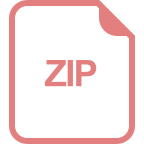
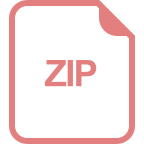
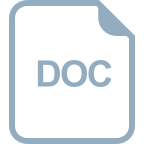
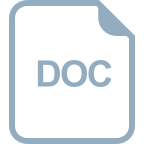
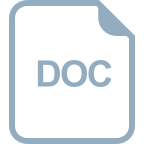
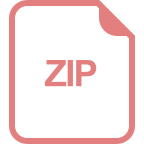