批量更新 mybatis
时间: 2023-11-16 08:55:28 浏览: 71
可以使用 MyBatis 的批量更新功能来提高更新效率。具体实现方式如下:
1. 在 Mapper.xml 文件中编写批量更新语句,例如:
```
<update id="batchUpdate" parameterType="java.util.List">
update user set name=#{name}, age=#{age} where id=#{id}
</update>
```
2. 在 Java 代码中调用批量更新方法,例如:
```
List<User> userList = new ArrayList<>();
// 添加需要更新的数据到 userList 中
sqlSession.update("batchUpdate", userList);
sqlSession.commit();
```
注意:在调用批量更新方法之前,需要先开启事务,并在更新完成后提交事务。
相关问题
Java批量更新mybatis
Java中使用MyBatis进行批量更新有两种常见的方式:
1. 直接在SQL语句中使用foreach循环进行批量更新,这种方式需要注意SQL语句的书写和参数的传递,示例代码如下:
```java
public void batchUpdate(List<Map<String, Object>> updateList) {
sqlSession.update("mapper.updateBatch", updateList);
}
```
其中,mapper.updateBatch对应的SQL语句为:
```sql
<update id="updateBatch" parameterType="java.util.List">
<foreach collection="list" item="item" index="index" separator=";">
update table_name set column1 = #{item.column1}, column2 = #{item.column2} where id = #{item.id}
</foreach>
</update>
```
2. 将需要更新的数据循环调用update方法去更新数据,示例代码如下:
```java
public void batchUpdate(List<Map<String, Object>> updateList) {
updateList.stream().forEach(map -> {
sqlSession.update("mapper.update", map);
});
}
```
其中,mapper.update对应的SQL语句为:
```sql
<update id="update" parameterType="java.util.Map">
update table_name set column1 = #{column1}, column2 = #{column2} where id = #{id}
</update>
```
springboot批量更新 mybatis
在SpringBoot中,使用MyBatis进行批量更新操作可以通过以下步骤实现:
1. 首先,确保你的项目中已经引入了相应的依赖,包括SpringBoot Starter和MyBatis-Plus的依赖。
2. 在配置文件中,设置MyBatis的相关规则,包括配置文件位置和Mapper接口对应的XML文件位置。
3. 在Mapper接口中定义相应的方法,用于批量更新操作。可以使用MyBatis-Plus提供的方法进行批量更新,例如updateBatchById方法。具体的更新逻辑可以根据业务需求自行编写。
4. 在业务层中调用Mapper接口的批量更新方法,传入需要更新的数据。
阅读全文
相关推荐
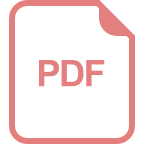
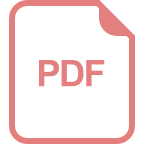
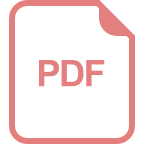












