用Java生成一个接入AnytingLLM的API的问答程序
时间: 2024-11-25 21:17:47 浏览: 11
要创建一个Java程序,用于通过API接入Anyting LLM(假设是一个类似ChatGPT的平台),你需要按照以下步骤进行:
1. **添加依赖**:
首先,确保你在项目中添加了处理HTTP请求的库,如`java.net`或第三方库,如Apache HttpClient、OkHttp或Spring Web。
```java
// 使用HttpURLConnection示例
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
// 或者使用第三方库,例如:
// import okhttp3.OkHttpClient;
```
2. **设置基础URL**:
定义YourAPIURL变量存储Anyting LLM的API地址,通常包括协议(http/https)、域名和路径。
```java
String YourAPIURL = "https://api.anytingllm.com/questions/";
```
3. **编写发送请求的方法**:
创建一个方法,它接受用户输入的问题作为参数,构造请求,并获取响应。
```java
public String sendQuestion(String question) throws Exception {
URL url = new URL(YourAPIURL + "?query=" + URLEncoder.encode(question, "UTF-8"));
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setRequestProperty("Content-Type", "application/json; charset=UTF-8");
int responseCode = connection.getResponseCode();
if (responseCode != HttpURLConnection.HTTP_OK) {
throw new RuntimeException("Failed : HTTP error code : " + responseCode);
}
BufferedReader br = new BufferedReader(new InputStreamReader((connection.getInputStream())));
StringBuilder response = new StringBuilder();
String output;
while ((output = br.readLine()) != null) {
response.append(output).append("\n");
}
br.close();
return response.toString();
}
```
4. **解析并返回答案**:
根据API的响应格式,解析数据并提取答案部分。这可能需要JSON解析库,如Jackson或Gson。
5. **测试和运行**:
创建一个主函数,接收用户输入,调用sendQuestion方法,然后打印结果。
```java
public static void main(String[] args) {
try {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入问题:");
String userQuestion = scanner.nextLine();
String answer = sendQuestion(userQuestion);
System.out.println("Anyting LLM的回答是:");
System.out.println(answer);
} catch (Exception e) {
System.err.println("发生错误: " + e.getMessage());
}
}
```
阅读全文
相关推荐




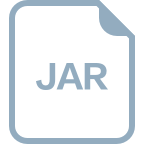











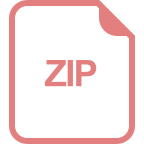
