给出c++实现的代码
时间: 2024-04-24 22:26:00 浏览: 128
以下是一个简单的示例代码,展示了如何在C++中加载和运行使用TensorRT转换的Yolov8 Pose模型:
```cpp
// 包含必要的头文件
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
#include <opencv2/opencv.hpp>
#include "NvInfer.h"
#include "NvOnnxParser.h"
// 定义一些常量
const int INPUT_H = 416;
const int INPUT_W = 416;
const int NUM_CLASSES = 80;
const int BATCH_SIZE = 1;
// 定义TensorRT的推理引擎和上下文
nvinfer1::IRuntime* runtime;
nvinfer1::ICudaEngine* engine;
nvinfer1::IExecutionContext* context;
// 加载TensorRT模型
void loadModel(const std::string& enginePath) {
std::ifstream engineFile(enginePath, std::ios::binary);
if (!engineFile) {
std::cerr << "无法打开TensorRT引擎文件!" << std::endl;
exit(1);
}
engineFile.seekg(0, std::ios::end);
size_t fileSize = engineFile.tellg();
engineFile.seekg(0, std::ios::beg);
std::vector<char> engineData(fileSize);
engineFile.read(engineData.data(), fileSize);
engineFile.close();
runtime = nvinfer1::createInferRuntime(gLogger);
engine = runtime->deserializeCudaEngine(engineData.data(), fileSize);
context = engine->createExecutionContext();
}
// 在图像上运行推理
void runInference(const cv::Mat& image) {
// 创建输入和输出缓冲区
float* inputBuffer;
float* outputBuffer;
cudaMalloc(&inputBuffer, BATCH_SIZE * 3 * INPUT_H * INPUT_W * sizeof(float));
cudaMalloc(&outputBuffer, BATCH_SIZE * NUM_CLASSES * sizeof(float));
// 对输入图像进行预处理
cv::Mat resizedImage;
cv::resize(image, resizedImage, cv::Size(INPUT_W, INPUT_H));
resizedImage.convertTo(resizedImage, CV_32F, 1 / 255.0);
float* inputData = reinterpret_cast<float*>(resizedImage.data);
// 将输入数据拷贝到GPU缓冲区中
cudaMemcpy(inputBuffer, inputData, BATCH_SIZE * 3 * INPUT_H * INPUT_W * sizeof(float), cudaMemcpyHostToDevice);
// 运行推理
void* buffers[] = { inputBuffer, outputBuffer };
context->executeV2(buffers);
// 获取输出结果
float* outputData = new float[NUM_CLASSES];
cudaMemcpy(outputData, outputBuffer, BATCH_SIZE * NUM_CLASSES * sizeof(float), cudaMemcpyDeviceToHost);
// 处理输出结果
for (int i = 0; i < NUM_CLASSES; ++i) {
std::cout << "类别 " << i << " 的概率:" << outputData[i] << std::endl;
}
// 释放资源
delete[] outputData;
cudaFree(inputBuffer);
cudaFree(outputBuffer);
}
int main() {
// 加载模型
std::string enginePath = "path/to/your/engine.plan";
loadModel(enginePath);
// 加载图像
std::string imagePath = "path/to/your/image.jpg";
cv::Mat image = cv::imread(imagePath);
// 运行推理
runInference(image);
// 释放资源
context->destroy();
engine->destroy();
runtime->destroy();
return 0;
}
```
请注意,上述代码仅为示例,可能需要根据您的实际情况进行适当的修改和调整。您需要根据您的环境和TensorRT版本进行相应的配置和编译。此外,还需要确保您已正确安装OpenCV,并提供正确的模型文件路径和图像文件路径。
希望这能帮助到您!如果您有任何问题,请随时提问。
阅读全文
相关推荐

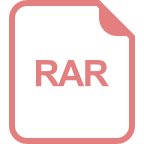
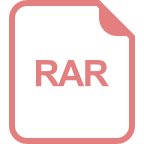
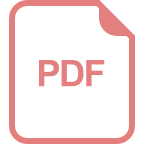




