算法与数据结构 校园导航资讯课程设计 Python
时间: 2024-12-31 08:23:53 浏览: 9
### 关于算法与数据结构的校园导航资讯课程设计
在进行基于Python的算法与数据结构应用于校园导航信息系统的设计时,可以考虑以下几个方面:
#### 数据存储与管理
为了有效地处理和查询地理空间信息,通常会采用树形结构来表示地图上的节点关系。例如,使用邻接表或邻接矩阵来描述路径连接情况[^1]。
```python
class Node:
def __init__(self, id):
self.id = id
self.neighbors = []
def add_edge(node_from, node_to):
node_from.neighbors.append(node_to)
```
#### 路径规划算法实现
A* (A-star) 是一种广泛使用的启发式搜索算法,在解决最短路径问题上表现优异。该算法通过估计从当前点到目标点的成本来进行优先级排序,从而更快找到最优解[^2]。
```python
import heapq
def heuristic(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def astar_search(start, goal):
frontier = []
heapq.heappush(frontier, (0, start))
came_from = {}
cost_so_far = {}
came_from[start] = None
cost_so_far[start] = 0
while frontier:
_, current = heapq.heappop(frontier)
if current == goal:
break
for next_node in current.neighbors:
new_cost = cost_so_far[current] + 1
if next_node not in cost_so_far or new_cost < cost_so_far[next_node]:
cost_so_far[next_node] = new_cost
priority = new_cost + heuristic(goal, next_node.position)
heapq.heappush(frontier, (priority, next_node))
came_from[next_node] = current
path = []
# Reconstruct the shortest path from 'came_from'
...
```
#### 用户界面开发
对于前端展示部分,可以选择Flask框架搭建Web应用,并利用Leaflet.js库提供交互式的地图视图功能。
阅读全文
相关推荐





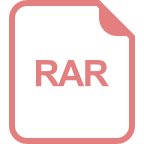
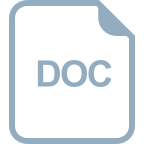
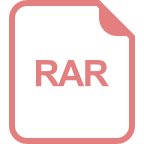

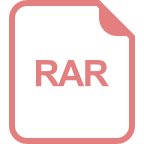








