基于图像处理和深度学习的齿轮表面缺陷检测的python代码
时间: 2024-12-24 18:22:07 浏览: 7
基于图像处理和深度学习进行齿轮表面缺陷检测的Python代码通常涉及以下几个步骤:
1. **数据预处理**:
```python
import cv2
from sklearn.model_selection import train_test_split
# 加载齿轮图像数据集
data = ... # 图像文件路径列表,标签列表
X, y = data['images'], data['labels']
# 数据增强,如旋转、缩放等
augmenter = imgaug.augmenters.Sequential([
imgaug.augmenters.Fliplr(0.5), # 水平翻转
imgaug.augmenters.RandomCrop((img_height, img_width)) # 随机裁剪
])
X_train, X_val, y_train, y_val = train_test_split(X, y, test_size=0.2, random_state=42)
X_train = augmenter(images=X_train)
# 转换为灰度图或预训练模型所需的输入格式
X_train_gray = np.array([cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) for img in X_train])
```
2. **特征提取**:
```python
from tensorflow.keras.applications.vgg16 import VGG16, preprocess_input
# 使用预训练模型提取特征
vgg16 = VGG16(weights='imagenet', include_top=False, input_shape=(img_height, img_width, 1))
features = vgg16.predict(X_train_gray)
features = features.reshape((-1, features.shape[-1]))
```
3. **构建深度学习模型**:
```python
from tensorflow.keras.layers import Dense, GlobalAveragePooling2D
from tensorflow.keras.models import Model
base_model = vgg16
x = base_model.output
x = GlobalAveragePooling2D()(x)
predictions = Dense(num_classes, activation='softmax')(x)
model = Model(inputs=base_model.input, outputs=predictions)
for layer in base_model.layers:
layer.trainable = False
# 冻结基础模型层,只训练添加的自定义层
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
```
4. **模型训练**:
```python
history = model.fit(X_train, y_train, validation_data=(X_val, y_val), epochs=num_epochs, batch_size=batch_size)
```
5. **评估与预测**:
```python
score = model.evaluate(X_test_gray, y_test)
print(f"Test accuracy: {score[1]}")
def predict_defect(image_path):
image = ... # 读取测试图像并转换为适当格式
prediction = model.predict(image)
return prediction
```
阅读全文
相关推荐
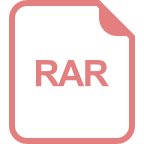
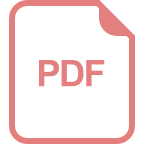
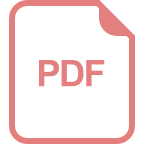
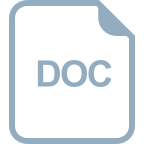
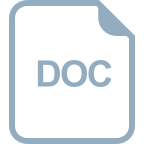
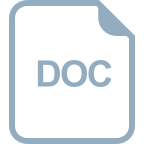
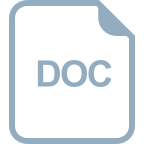
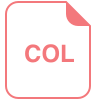
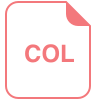
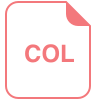
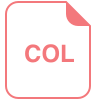
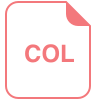
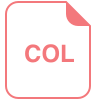
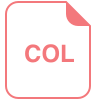
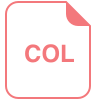
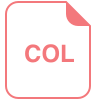
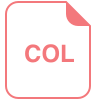
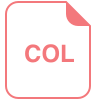
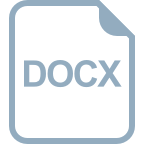