ADMMpython
时间: 2024-12-27 16:23:54 浏览: 6
### ADMM Implementation in Python
In the context of implementing Alternating Direction Method of Multipliers (ADMM) within Python, a structured approach is necessary to ensure that both efficiency and accuracy are maintained during optimization problems. The method involves decomposing complex optimization tasks into simpler subproblems which can be solved iteratively.
The core idea behind ADMM lies in breaking down an original problem into smaller parts by introducing auxiliary variables along with constraints linking these new variables back to the originals[^3]. This allows for parallel processing capabilities as well as easier handling of non-differentiable terms or large datasets.
Below demonstrates how one might implement basic ADMM functionality using NumPy:
```python
import numpy as np
def admm(x_init, A, b, rho=1., max_iter=100):
"""
Solves Ax=b via ADMM.
Parameters:
x_init : Initial guess for solution vector 'x'.
A : Coefficient matrix from linear system equation "Ax = b".
b : Right-hand side column vector from linear system equation "Ax = b".
rho : Step size parameter used within updates; defaults to 1.
max_iter: Maximum number of iterations before stopping criterion met; default set at 100.
Returns:
Tuple containing final value of primal variable `x`, dual variable `z`
and Lagrange multiplier `u`.
"""
m, n = A.shape
# Initialize variables
x = x_init.copy()
z = np.zeros((n,))
u = np.zeros_like(z)
AtA = A.T @ A
inv_AAtI = np.linalg.inv(AtA + rho * np.eye(n))
for _ in range(max_iter):
# Update rule for primary variable x
x_new = inv_AAtI @ (A.T @ b + rho * (z - u))
# Soft thresholding operation applied on updated version of secondary variable z
soft_threshold = lambda v, k: np.sign(v)*np.maximum(abs(v)-k, 0.)
z_new = soft_threshold(x_new + u, 1./rho)
# Dual ascent step updating lagrangian multipliers u
u += x_new - z_new
# Check convergence criteria here if needed...
# Prepare next iteration values
x[:] = x_new[:]
z[:] = z_new[:]
return x, z, u
```
This code snippet provides a simple yet effective way to apply ADMM towards solving least squares type equations represented through matrices \( \mathbf{A} \), vectors **b**, alongside initial guesses provided for unknowns denoted by **x**. Note this example uses Lasso regression's penalty term inside its update rules but could easily adapt depending upon specific application requirements.
阅读全文
相关推荐
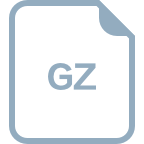







