用c语言创建一个允许n个进程并发运行的进程管理模拟系统
时间: 2024-10-09 12:07:43 浏览: 55
在C语言中创建一个进程管理模拟系统,允许N个进程并发运行,通常涉及到信号量(Semaphore)、互斥锁(Mutex)等同步原语,因为C标准库提供的功能并不直接支持多线程,我们通常使用线程库如pthread。下面是一个简单的示例,使用信号量来控制资源访问:
```c
#include <stdio.h>
#include <stdlib.h>
#include <semaphore.h> // 包含信号量头文件
#define MAX_PROCESSES 5 // 最大进程数
#define RESOURCES 3 // 共享资源数量
// 信号量初始化
sem_t resources, mutex;
void *process(void* arg) {
int id = *(int*)arg;
sem_wait(&resources); // 获取资源
printf("Process %d started, got resource.\n", id);
// 这里模拟处理任务...
sleep(2); // 模拟任务处理时间
printf("Process %d finished, releasing resource.\n", id);
sem_post(&resources); // 释放资源
return NULL;
}
int main() {
if (sem_init(&resources, 0, RESOURCES) == -1) {
perror("Semaphore initialization failed");
exit(EXIT_FAILURE);
}
if (sem_init(&mutex, 0, 1) == -1) { // 初始化互斥锁
perror("Mutex initialization failed");
exit(EXIT_FAILURE);
}
pthread_t processes[MAX_PROCESSES];
for (int i = 0; i < MAX_PROCESSES; ++i) {
int pid = i + 1;
if (pthread_create(&processes[i], NULL, process, &pid) != 0) {
perror("Thread creation failed");
exit(EXIT_FAILURE);
}
}
for (int i = 0; i < MAX_PROCESSES; ++i) {
if (pthread_join(processes[i], NULL) != 0) {
perror("Thread joining failed");
exit(EXIT_FAILURE);
}
}
sem_destroy(&resources);
sem_destroy(&mutex);
printf("All processes completed.\n");
return 0;
}
阅读全文
相关推荐
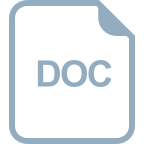
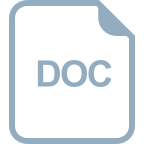
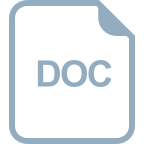

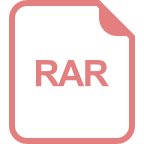
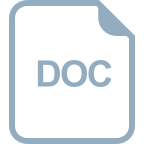
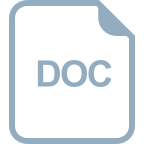











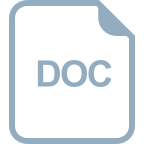