c#中有两个数组,想把这两个数组合并成一个数组并且排序怎么写
时间: 2024-10-25 17:04:54 浏览: 25
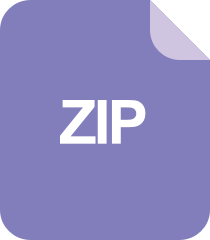
c#-Leetcode面试题解之第4题寻找两个正序数组的中位数.zip
在C#中,你可以使用`List<T>`或者`Array`类的静态方法来合并并排序两个数组。这里我们假设两个数组都是同类型的,并且可以安全地进行比较和排序。
以下是使用`List<T>`的例子:
```csharp
using System;
using System.Collections.Generic;
class Program
{
static void Main()
{
// 假设你有两个整数数组
int[] array1 = { 5, 2, 7 };
int[] array2 = { 3, 9, 1 };
// 合并到一个新的List中
List<int> combinedList = new List<int>(array1);
combinedList.AddRange(array2);
// 对List进行排序
combinedList.Sort();
// 如果需要转换回数组,可以这样做
int[] sortedArray = combinedList.ToArray();
Console.WriteLine("Sorted Array:");
foreach (int num in sortedArray)
{
Console.Write(num + " ");
}
}
}
```
如果你坚持使用数组,你可以先将它们复制到新的数组,然后使用`Array.Sort()`方法:
```csharp
using System;
class Program
{
static void Main()
{
int[] array1 = { 5, 2, 7 };
int[] array2 = { 3, 9, 1 };
// 创建一个新的数组来保存结果
int[] combinedAndSortedArray = new int[array1.Length + array2.Length];
// 将第一个数组复制到新数组
Array.Copy(array1, 0, combinedAndSortedArray, 0, array1.Length);
// 将第二个数组添加到新数组
Array.Copy(array2, 0, combinedAndSortedArray, array1.Length, array2.Length);
// 对新数组进行排序
Array.Sort(combinedAndSortedArray);
// 打印排序后的数组
for (int i = 0; i < combinedAndSortedArray.Length; i++)
{
Console.Write(combinedAndSortedArray[i] + " ");
}
}
}
```
阅读全文
相关推荐
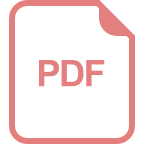
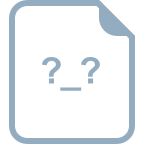


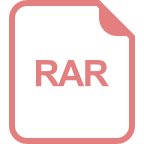
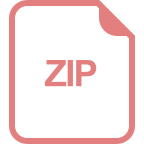
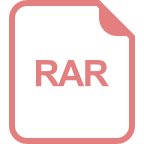
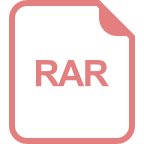
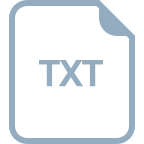
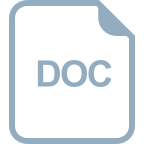
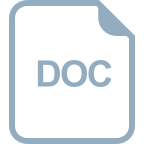
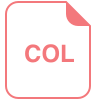





