如何使用内联汇编检测线程栈的空间使用情况,请列举16位、32位、64位寄存器的c++代码来说明
时间: 2024-09-13 22:07:32 浏览: 56
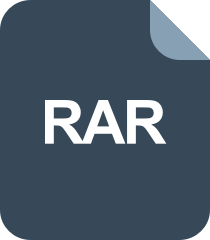
在 Visual C++ 中使用内联汇编,VC内联ASM汇编,混合使用C和汇编代码的程序(用__asm写c函数)
使用内联汇编检测线程栈空间使用情况通常是为了获取程序运行时栈信息,以便了解其内存消耗。以下是一个简化的示例,展示了如何在16位、32位和64位平台下使用C++和内联汇编来检查栈指针的变化:
**16位平台 (x86) - Intel i386**
```cpp
#include <iostream>
#include <asm>
void printStackUsage() {
__asm__ volatile (
"pushl %esp" // Save the stack pointer before incrementing it
"addl $4, %esp" // Increment the stack pointer by a small amount (e.g., sizeof(int))
"popl %esp" // Restore the original stack pointer
: "=m"(*__esp) // Store the new stack value in a variable
: // No input operands
: "%eax", "%ebx" // Clobber registers that may be used
);
std::cout << "Current stack usage: " << *(__esp) << " bytes\n";
}
int main() {
int i;
printStackUsage();
return 0;
}
```
**32位平台 (x86-64 or ARM)**
```cpp
#include <iostream>
#include <asm/x86_64>
void printStackUsage() {
__asm__ volatile (
".byte 0x55" // Push RIP and mov RBP to stack
"pushq %rsp"
"subq $4, %rsp" // Allocate space for an integer
"movl $0, (%rsp)" // Place an int at the stack top
"popq %rbp" // Restore RBP
"leave"
"movq %rsp, %rax" // Read current stack pointer
: "=r"(stack_usage)
: // No input operands
: "%rax", "%rbp", "%rsp"
);
std::cout << "Current stack usage: " << stack_usage << " bytes\n";
}
int main() {
int i;
printStackUsage();
return 0;
}
```
**64位平台 (x86-64 or x86_64)**
```cpp
#include <iostream>
#include <asm/x86_64>
void printStackUsage() {
__asm__ volatile (
"pushq %rsp" // Save RSP
"subq $8, %rsp" // Allocate space for an integer and align
"movl $0, [rip+0]" // Place an int at the stack top using RIP-relative addressing
"popq %rsp" // Restore RSP
"movq %rsp, %rax" // Read current stack pointer
: "=r"(stack_usage)
: // No input operands
: "%rax", "%rsp"
);
std::cout << "Current stack usage: " << stack_usage << " bytes\n";
}
int main() {
int i;
printStackUsage();
return 0;
}
```
注意:实际应用中,直接访问堆栈可能会有安全风险,并且依赖于特定架构和编译选项。上述代码仅作教学示例,并非生产环境推荐实践。
阅读全文
相关推荐
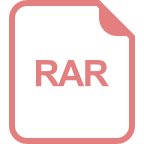
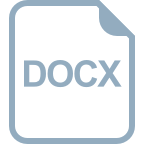
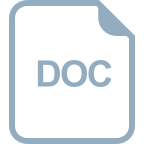
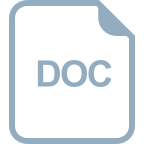
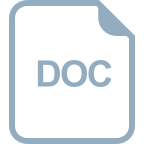
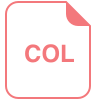
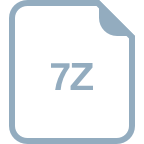
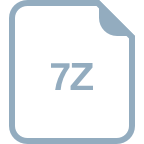