mybatis子查询写法
时间: 2023-11-07 16:16:05 浏览: 239
在 MyBatis 中,你可以使用子查询来实现复杂的查询逻辑。下面是一个示例,展示了 MyBatis 中如何使用子查询:
```xml
<!-- 定义一个查询语句 -->
<select id="getParents" resultType="Parent">
SELECT id, name
FROM parents
WHERE id IN (
<!-- 子查询 -->
SELECT parent_id
FROM children
WHERE age > #{age}
)
</select>
```
在上面的示例中,我们有两个表:parents 和 children。我们想要查询在 children 表中年龄大于给定值 age 的所有父母。这里使用了一个子查询来获取满足条件的父母的 ID,并将其用作外部查询的过滤条件。
你可以根据自己的需求来修改这个示例,并将其应用到你的具体情况中。希望对你有所帮助!如果你有更多问题,可以继续提问。
相关问题
mybatis plus sql in写法
MyBatis-Plus 提供了 `in` 查询的多种写法,以下是几种常用的方式:
1. 使用 `in` 方法:通过 `in` 方法可以传入一个集合或数组作为查询条件。
```java
List<Integer> ids = Arrays.asList(1, 2, 3);
List<User> userList = userMapper.selectList(new QueryWrapper<User>().in("id", ids));
```
2. 使用 `inSql` 方法:通过 `inSql` 方法可以传入一个 SQL 子查询作为查询条件。
```java
String subQuery = "SELECT id FROM user WHERE age > 18";
List<User> userList = userMapper.selectList(new QueryWrapper<User>().inSql("id", subQuery));
```
3. 使用 lambda 表达式:可以直接使用 lambda 表达式构建 `in` 查询条件。
```java
List<Integer> ids = Arrays.asList(1, 2, 3);
List<User> userList = userMapper.selectList(Wrappers.<User>lambdaQuery().in(User::getId, ids));
```
4. 使用条件构造器:可以使用条件构造器来动态构建 `in` 查询条件。
```java
QueryWrapper<User> queryWrapper = new QueryWrapper<>();
queryWrapper.in("id", 1, 2, 3);
List<User> userList = userMapper.selectList(queryWrapper);
```
以上是一些常见的 MyBatis-Plus 中 `in` 查询的写法,你可以根据具体的场景选择适合的方法。
select <foreach collection="columnNameList" separator="," item="columnName" close=","> ${columnName} </foreach> a."bridge_code" as "system_code" from ${tableName} left join ( select hbmdt.code as "point_code", hbmdt.collect_code as "collect_code", hbmtt.bridge_code as "bridge_code" from "hm_bridge_monitor_device_tbl" hbmdt left join "hm_bridge_monitor_type_tbl" hbmtt on hbmdt.monitor_type = hbmtt.monitor_type ) as "a" on ${tableName}.point_code = a."point_code" or ${tableName}.collect_code = a.collect_code order by time desc limit 3000 offset 0 将这段mybatissql转换城jooq写法
以下是将该 MyBatis SQL 转换为 JOOQ 写法的代码:
```java
import static org.jooq.impl.DSL.*;
import org.jooq.*;
import org.jooq.impl.*;
public class MyMapper {
public Result<Record> myQuery(DSLContext ctx, String tableName, String[] columnNameList) {
Table<?> a = table(
select(
field(name("hbmdt", "code")).as("point_code"),
field(name("hbmdt", "collect_code")).as("collect_code"),
field(name("hbmtt", "bridge_code")).as("bridge_code")
)
.from(table(name("hm_bridge_monitor_device_tbl")).as("hbmdt"))
.leftJoin(table(name("hm_bridge_monitor_type_tbl")).as("hbmtt"))
.on(field(name("hbmdt", "monitor_type")).eq(field(name("hbmtt", "monitor_type"))))
).as("a");
SelectConditionStep<Record> select = ctx.select(
field(name("a", "bridge_code")).as("system_code")
);
for (String columnName : columnNameList) {
select = select.select(field(name(columnName)));
}
select = select.from(table(name(tableName)))
.leftJoin(a)
.on(field(name(tableName, "point_code")).eq(field(name("a", "point_code")))
.or(field(name(tableName, "collect_code")).eq(field(name("a", "collect_code")))))
.orderBy(field(name("time")).desc())
.limit(3000)
.offset(0);
return select.fetch();
}
}
```
需要注意的是,由于该 SQL 中的列名和表名都是动态的,因此需要将它们作为参数传入方法中。在 JOOQ 中,使用 `name()` 方法将字符串转换为 SQL 名称。由于 `select` 子句中的列名也是动态的,因此需要动态构建 `select` 子句。在 JOOQ 中,可以通过连续调用 `select()` 方法来动态添加列。在 JOIN 子句中,可以使用 `table()` 方法将子查询转换为一个可用的表,然后使用 `as()` 方法为其命名。在 ON 子句中,可以使用 `field()` 方法获取字段,然后使用 `eq()` 方法进行比较。在 ORDER BY 子句中,可以使用 `orderBy()` 方法指定排序列和排序方向。最后,使用 `limit()` 和 `offset()` 方法来指定查询的行数和偏移量,然后使用 `fetch()` 方法执行查询并返回结果。
阅读全文
相关推荐
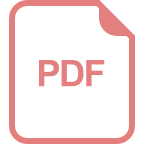
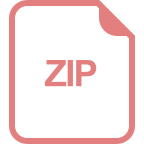
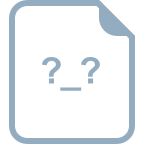
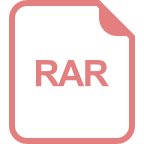
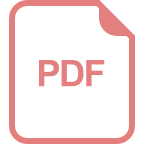
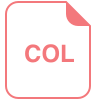
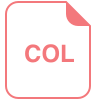
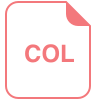
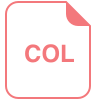

