写一段用printf输入 scanf检索输入数据的代码
时间: 2024-10-02 21:01:22 浏览: 33
当然可以,这里是一个简单的示例,展示了如何使用`printf`提示用户输入数据,然后使用`scanf`获取这些输入并在控制台上打印出来:
```c
#include <stdio.h> // 包含printf和scanf头文件
int main() {
int input; // 定义一个整型变量来保存用户的输入
printf("请输入一个整数: "); // 使用printf显示提示信息
// 使用scanf读取用户的输入并存储在input中
if (scanf("%d", &input) == 1) { // 如果输入有效,返回值为1
printf("您输入的数字是: %d\n", input); // 打印用户输入的数值
} else {
printf("无效的输入,请输入一个整数。\n"); // 输入不是数字的情况
}
return 0;
}
```
在这个例子中,`printf`用于提示用户输入,`scanf`负责读取用户的输入并将其转化为整数。如果输入是有效的,程序会继续执行并打印出用户输入的值;否则,会给出错误提示。
相关问题
可以用printf和scanf写一个程序吗?
当然可以。在C语言中,`printf()` 和 `scanf()` 是两个常用的库函数,分别用于输出和读取数据。它们属于标准输入输出库 `<stdio.h>`。
`printf()` 函数用于格式化输出字符串,常用于打印结果信息。例如,你可以编写这样的代码片段:
```c
#include <stdio.h>
int main() {
int number = 10;
char name[] = "Alice";
printf("The number is %d and the name is %s.\n", number, name); // 输出:The number is 10 and the name is Alice.
return 0;
}
```
而 `scanf()` 函数则用于从标准输入(通常是键盘)读取数据到变量中,例如:
```c
#include <stdio.h>
int main() {
int age;
float grade;
printf("Please enter your age: ");
scanf("%d", &age); // 用户输入年龄,存储到age变量
printf("Please enter your grade: ");
scanf("%f", &grade); // 用户输入分数,存储到grade变量
return 0;
}
```
这两个函数配合起来可以方便地进行用户交互和数据展示。
有多个printf和scanf,却只有第一个printf能输入
这可能是由于程序设计的问题或者是用户交互环境的一个特性。通常,在标准的控制台上,`printf` 和 `scanf` 函数会交替作用,`printf` 用于打印信息,而 `scanf` 用于从用户那里接收输入。然而,如果只有一个 `scanf` 被放在了可以接受用户输入的地方,那么确实只能获取一次输入。
有几个可能的原因:
1. **顺序执行**:如果 `printf` 和 `scanf` 没有通过循环或者其他机制配合,程序只会按照编写顺序依次运行,所以用户只能响应第一次的 `scanf`。
2. **缓冲区**:`scanf` 可能读取的是前一次的缓存输入,如果没有新的输入,它将返回空值。除非后续代码检查并处理这种情况。
3. **用户界面限制**:在某些终端模拟器或者IDE环境下,可能只允许一个标准输入流(如键盘),所以只能对一个 `scanf` 发出响应。
如果你遇到这个问题,解决办法通常是确保有足够的输入处理机制,或者使用循环来多次请求用户输入。如果你想让每个 `printf` 都能接收到输入,可以在适当的地方添加 `getchar()` 或者 `scanf("",&input)` 来清除缓存并等待新输入。
阅读全文
相关推荐
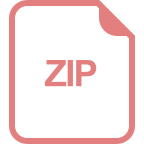
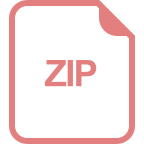
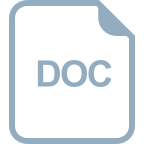













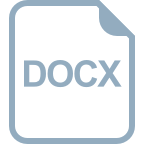