使用 gui.ava:html2image 库将 HTML 转换为图片,保存到本地,然后将图片转换为 Base64 字符串并通过 HTTP POST 请求发送到下游系统
时间: 2024-11-30 15:15:19 浏览: 17
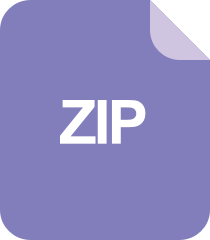
maven 下载到 maven库下边的 gui.ava.html2image0.9 及jar 可以直接使用
HTML2Image 是一个 Java 库,用于将 HTML 内容转换成图片。以下是使用此库的基本步骤:
1. **添加依赖**:首先,你需要在你的项目中添加 html2image 的依赖。如果你使用的是 Maven 或 Gradle 等构建工具,可以在构建文件中添加相应的库引用。
```xml
<!-- Maven 示例 -->
<dependency>
<groupId>com.github.html2image</groupId>
<artifactId>html2image</artifactId>
<version>0.9.5</version> <!-- 查看最新版本 -->
</dependency>
<!-- Gradle 示例 -->
implementation 'com.github.html2image:html2image:0.9.5'
```
2. **转换 HTML 到 Image**:
- 导入必要的类:
```java
import com.github.html2image.Html2Image;
```
- 创建 Html2Image 实例,并调用其 `convertToPng` 方法将 HTML 转换成 PNG 图片:
```java
String htmlContent = "<your_html_here>";
Html2Image html2Image = new Html2Image();
byte[] imageBytes = html2Image.convertToPng(htmlContent);
```
3. **保存图片到本地**:
- 你可以使用 Java 的 File 类来存储图片:
```java
try (FileOutputStream fos = new FileOutputStream("output.png")) {
fos.write(imageBytes);
} catch (IOException e) {
e.printStackTrace();
}
```
4. **将图片转为 Base64**:
- 使用 java.util.Base64 对字节数组编码:
```java
String base64Image = Base64.getEncoder().encodeToString(imageBytes);
```
5. **发送 HTTP POST 请求**:
- 使用 Java 的 HttpURLConnection 或者第三方库如 Apache HttpClient 发送 POST 请求,附带 Base64 编码后的图像数据:
```java
URL url = new URL("http://downstream-api.com/upload");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setRequestProperty("Content-Type", "application/octet-stream");
OutputStream os = connection.getOutputStream();
os.write(base64Image.getBytes(StandardCharsets.UTF_8));
// Close resources and handle response.
```
记得处理可能出现的异常,并在请求完成后关闭连接。
阅读全文
相关推荐
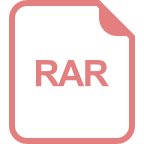
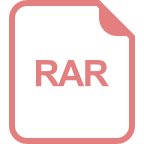
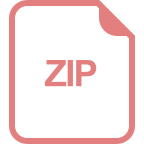
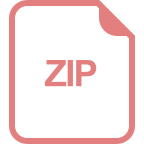
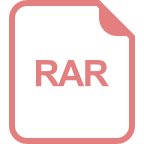
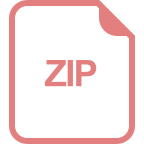
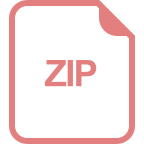
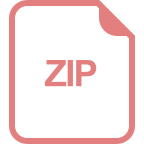
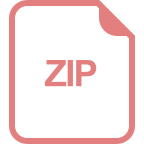
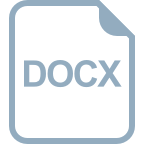
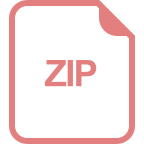
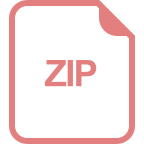
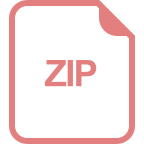
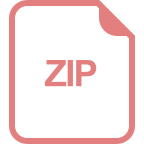
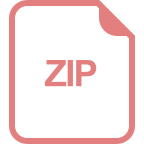
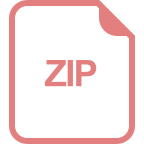
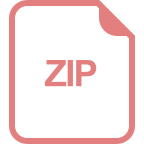
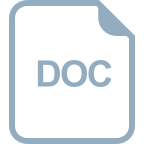