vue 获取两个时间戳差值
时间: 2024-10-12 13:06:04 浏览: 67
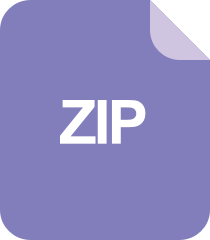
vue获取当前时间.zip
在Vue中,如果你需要获取两个时间戳之间的差值(通常以毫秒或秒为单位),你可以使用JavaScript内置的Date对象和一些简单的数学计算。这里是一个例子:
```javascript
new Date().getTime() - anotherTimestamp // where anotherTimestamp是你另一个时间戳的变量
// 或者如果你有两个Date对象
const date1 = new Date(timestamp1);
const date2 = new Date(timestamp2);
const diffInMilliseconds = Math.abs(date2.getTime() - date1.getTime());
```
`getTime()`方法会返回一个表示自1970年1月1日 00:00:00 UTC以来的毫秒数,所以相减就可以得到两个时间点之间的时间差。
如果你想将结果转换成更易读的形式,如分钟或小时,可以除以相应的转换因子(例如60,000 for milliseconds to seconds,3,600,000 for seconds to minutes等)。
阅读全文
相关推荐
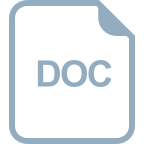
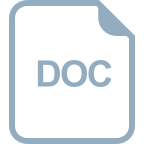
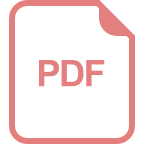
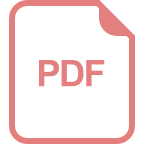
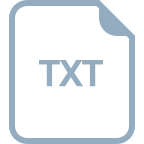
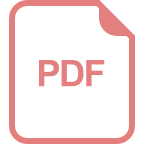
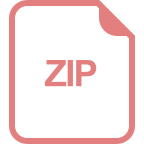



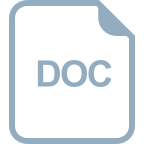
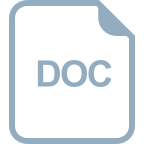
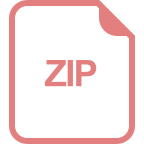
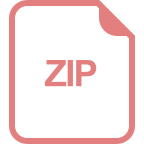
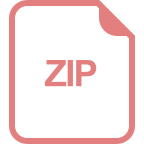
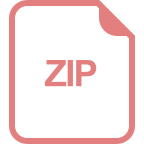
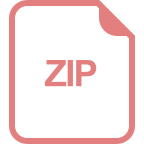