C#抽象方法与虚方法
时间: 2023-11-23 17:17:07 浏览: 76
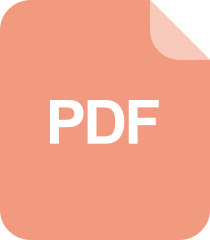
C#中的虚方法和抽象方法的运用
抽象方法和虚方法都是面向对象编程中的重要概念,用于实现多态性和继承。
抽象方法(Abstract Method)是在抽象类或接口中声明的方法,它没有具体的实现代码。抽象方法只有方法的声明,而没有方法体。抽象方法必须在非抽象子类中被实现,否则该子类也必须声明为抽象类。使用抽象方法可以强制子类实现特定的方法,以满足特定的需求。在C#中,使用`abstract`关键字来声明抽象方法。
示例代码:
```csharp
public abstract class Animal {
public abstract void MakeSound();
}
public class Dog : Animal {
public override void MakeSound() {
Console.WriteLine("Woof!");
}
}
public class Cat : Animal {
public override void MakeSound() {
Console.WriteLine("Meow!");
}
}
Animal dog = new Dog();
dog.MakeSound(); // 输出: Woof!
Animal cat = new Cat();
cat.MakeSound(); // 输出: Meow!
```
虚方法(Virtual Method)是在基类中声明的可以被子类重写的方法。虚方法提供了一种方式,让子类可以根据自己的需求来重新定义基类中的行为。在C#中,使用`virtual`关键字来声明虚方法。
示例代码:
```csharp
public class Shape {
public virtual void Draw() {
Console.WriteLine("Drawing a shape.");
}
}
public class Circle : Shape {
public override void Draw() {
Console.WriteLine("Drawing a circle.");
}
}
public class Square : Shape {
public override void Draw() {
Console.WriteLine("Drawing a square.");
}
}
Shape shape = new Circle();
shape.Draw(); // 输出: Drawing a circle.
shape = new Square();
shape.Draw(); // 输出: Drawing a square.
```
在上述示例中,基类`Shape`中的`Draw`方法被声明为虚方法,子类`Circle`和`Square`可以根据自己的需求来重写`Draw`方法,实现不同的绘制行为。
阅读全文
相关推荐


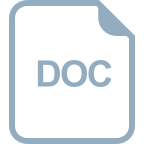
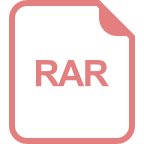
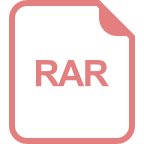
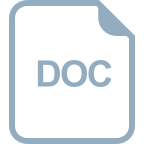
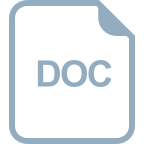
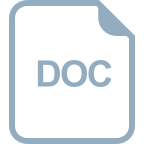
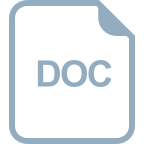

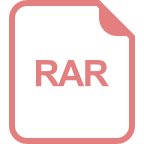
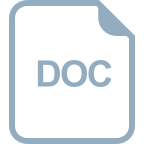
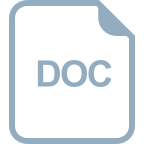
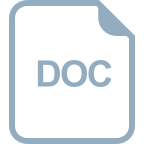
