Python密码校验
时间: 2024-11-28 16:11:50 浏览: 0
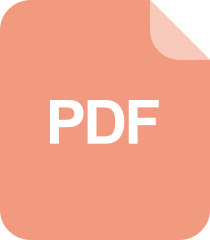
python实现密码强度校验
在Python中,密码校验通常涉及到对用户输入的密码进行一些基本验证,如长度、字符种类、是否包含特殊字符等。这可以通过正则表达式(regex)或内置函数实现。以下是一些常见的密码校验步骤:
1. **长度检查**:确认密码是否满足最小和最大长度要求。可以使用`len()`函数获取字符串长度。
```python
import re
MIN_LENGTH = 8
MAX_LENGTH = 16
def check_length(password):
return MIN_LENGTH <= len(password) <= MAX_LENGTH
```
2. **复杂度检查**:确保密码包含字母、数字和可能的特殊字符。可以使用正则表达式匹配模式。
```python
def has_complexity(password):
pattern = r"^(?=.*[a-zA-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$"
return bool(re.match(pattern, password))
```
3. **强密码策略**:还可以设置特定的要求,比如至少一个大写字母、一个小写字母、一个数字和一个特殊字符。
```python
def strong_password(password):
has_uppercase = any(char.isupper() for char in password)
has_lowercase = any(char.islower() for char in password)
has_digit = any(char.isdigit() for char in password)
has_special = any(c in '!@#$%^&*()' for c in password)
return has_uppercase and has_lowercase and has_digit and has_special
```
阅读全文
相关推荐
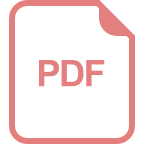
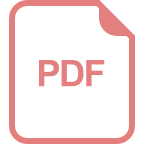
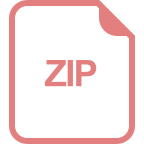
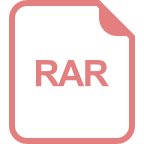
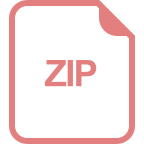









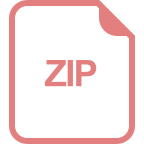
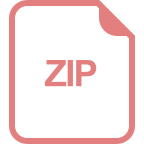