使用Springboot webclient发送post请求更新数据,要求更新的数据以param形式去发送请求,然后api返回的response的格式是xml形式的,如何接收,请写出完整的代码
时间: 2024-12-24 09:40:17 浏览: 2
在Spring Boot应用中,我们可以使用WebClient作为HTTP客户端发送POST请求,并将参数以`application/x-www-form-urlencoded`格式发送。对于XML格式的响应,我们需要配置一个XML解析器来解析服务器返回的内容。以下是一个简单的示例:
首先,引入必要的依赖:
```groovy
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
// 如果需要XML支持,可以添加这个
implementation 'com.fasterxml.jackson.dataformat:jackson-dataformat-xml'
}
```
然后,创建一个Service来处理WebClient操作:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.client.reactive.ReactorNettyHttpURLConnectionClient;
import org.springframework.stereotype.Service;
import org.springframework.web.reactive.function.BodyInserters;
import org.springframework.web.reactive.function.client.WebClient;
import reactor.core.publisher.Mono;
@Service
public class DataUpdateService {
private final WebClient webClient;
@Autowired
public DataUpdateService(ReactorNettyHttpURLConnectionClient client) {
this.webClient = WebClient.builder().clientConnector(client).build();
}
public Mono<Void> updateData(String url, Map<String, String> params) {
return webClient.post()
.uri(url)
.headers(h -> h.setContentType(MediaType.APPLICATION_FORM_URLENCODED))
.body(BodyInserters.fromValue(params))
.retrieve()
.bodyToMono(String.class);
}
// 如果API返回的是XML,你需要添加一个XML解析逻辑,例如:
public Mono<SomeXmlType> parseXmlResponse(String responseBody) {
return Mono.just(responseBody)
.map(xmlString -> mapper.readValue(xmlString, SomeXmlType.class));
}
private final ObjectMapper mapper = new ObjectMapper();
// ...在这里初始化一个ObjectMapper实例(如果Jackson XML模块已添加)
}
// 注意,SomeXmlType应该替换为你实际期望从XML中反序列化的实体类型
```
在这个例子中,`updateData`方法用于发送POST请求,`parseXmlResponse`方法用于将接收到的XML字符串转换为对应的Java对象。
阅读全文
相关推荐
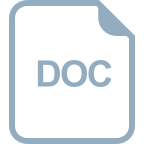
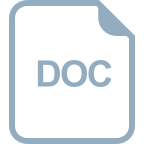
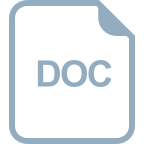

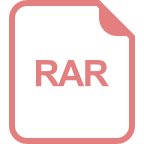
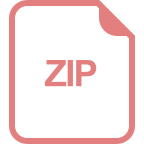
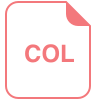



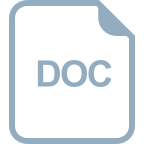
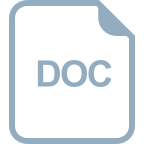
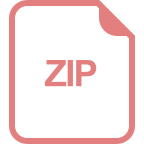