导线网间接平差计算程序
时间: 2024-12-28 16:29:37 浏览: 6
### 导线网间接平差计算程序实现方法
#### Python 实现导线网间接平差计算
为了实现导线网的间接平差,在Python中可以利用NumPy库来处理矩阵运算。以下是基于最小二乘法的一个简单例子,用于求解未知参数向量\( \hat{x} \),并最终得到改正数和精度估计。
```python
import numpy as np
def indirect_adjustment(A, l, P=None):
"""
A: 设计矩阵 (m×n)
l: 观测值向量 (m×1)
P: 权阵,默认为单位阵 (m×m)
返回:
v: 改正数向量 (m×1)
x_hat: 参数估值向量 (n×1)
sigma_0: 单位权方差
"""
m, n = A.shape
if P is None:
P = np.eye(m)
N = A.T @ P @ A # 法方程系数矩阵
b = A.T @ P @ l # 常数项列向量
try:
invN = np.linalg.inv(N) # 计算法方程逆矩阵
except np.linalg.LinAlgError:
raise ValueError('无法求得法方程逆矩阵')
x_hat = invN @ b # 解出参数估值
v = l - A @ x_hat # 计算改正数
VtPV = v.T @ P @ v # 后验单位权方差分母部分
r = m - n # 自由度
sigma_0 = np.sqrt(VtPV / r) # 计算单位权标准偏差
return {'v': v,
'x_hat': x_hat,
'sigma_0': sigma_0}
```
此函数实现了基本的间接平差过程[^1]。
#### MATLAB 实现导线网间接平调计算
对于MATLAB环境下的实现方式,则更加简洁明了:
```matlab
function [V,X,Sigma]=indirectAdjustment(A,l,P)
% 输入变量说明同上
if nargin<3 || isempty(P), P=eye(length(l)); end;
N=A'*P*A; % 构造正规化方程式左边
b=A'*P*l; % 构造右边常数项
X=N\b; % 求解未知数
V=l-A*X; % 得到残差
Sigma=sqrt((V'*P*V)/(length(l)-size(A,2))); % 计算单位权均方根误差
end
```
这段代码同样遵循了间接平差的核心思路,并且能够有效地完成相应的数值分析工作[^2]。
#### C++ 实现导线网间接平差计算
而在C++环境下,由于缺乏内置的支持科学计算的强大工具包,因此可能需要借助第三方库如Eigen来进行高效的数据操作:
```cpp
#include <iostream>
#include "Eigen/Dense"
using namespace std;
typedef Eigen::MatrixXd MatrixXd;
void Indirect_Adjustment(const MatrixXd& A, const VectorXd& L, double *sigma){
int rows = A.rows(), cols = A.cols();
MatrixXd AtPA = A.transpose() * A;
VectorXd AtPL = A.transpose() * L;
// Solve the normal equation system using LU decomposition.
VectorXd X = AtPA.fullPivLu().solve(AtPL);
// Calculate residual vector and its sum of squares weighted by precision matrix.
VectorXd V = L - A * X;
double sswr = V.dot(V);
// Compute unit weight standard deviation.
(*sigma)=sqrt(sswr/(rows-cols));
}
int main(){
/* Example usage */
MatrixXd A(4,2);VectorXd L(4);
// Fill matrices with your data...
double sigma;
Indirect_Adjustment(A,L,&sigma);
cout << "Unit Weight Standard Deviation:"<< sigma<< endl;
return 0;
}
```
上述三种编程语言都展示了如何通过构建设计矩阵A、观测值l以及可选权重矩阵P来执行间接平差的过程。
阅读全文
相关推荐
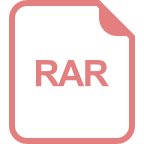
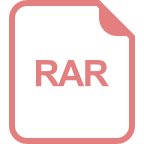
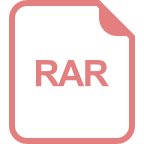
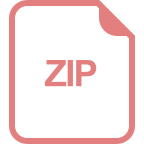


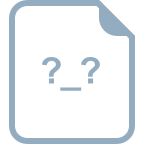
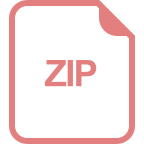
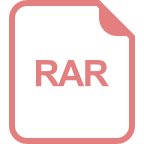
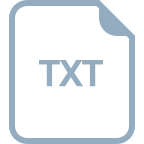
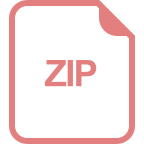
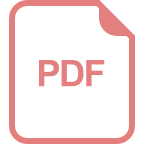
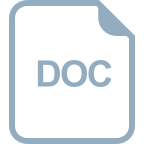
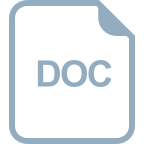


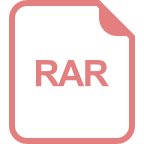
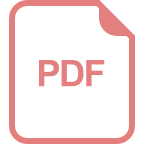
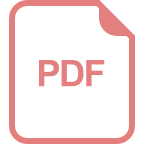