vue移除sessionstorage
时间: 2024-09-15 13:04:07 浏览: 36
在Vue.js中,如果你想从浏览器的`localStorage`或`sessionStorage`中移除某个键值对,你可以直接使用JavaScript的相应API。以下是移除`sessionStorage`中数据的例子:
```javascript
// 移除特定键的数据
function removeSessionData(key) {
if (typeof sessionStorage !== 'undefined') {
sessionStorage.removeItem(key);
}
}
// 如果你想移除整个存储,可以这样做
function clearSessionStorage() {
if (typeof sessionStorage !== 'undefined') {
sessionStorage.clear();
}
}
// 调用函数并传入你需要移除的键名
removeSessionData('yourKey'); // 或者 clearSessionStorage() 来清除所有数据
```
在Vue组件中,你可以将上述函数绑定到按钮的点击事件或其他需要的操作上。记得在实际应用中替换`'yourKey'`为你想要移除的具体键名。
相关问题
Vue监听sessionStorage数据
在Vue.js中,由于`sessionStorage`是浏览器原生的,并非Vue直接提供API来监听它的变化。不过,你可以结合浏览器提供的事件监听机制,再加上一些辅助函数,来实现类似的效果。这里是一个简单的示例,展示如何手动监听`sessionStorage`的变化:
```javascript
// 定义一个全局的watcher
const observeSessionStorage = (key, callback) => {
const previousValue = sessionStorage.getItem(key);
window.addEventListener('storage', (event) => {
if (event.key === key && event.newValue !== previousValue) {
callback(event.newValue);
previousValue = event.newValue;
}
});
};
// 在需要的地方使用
observeSessionStorage('yourKey', (newValue) => {
console.log(`New session storage value: ${newValue}`);
});
// 取消监听时
window.removeEventListener('storage', (event) => {
if (event.key === 'yourKey') {
// 移除监听或清理回调
}
});
```
请注意,这只是一个基本的实现,实际应用中可能需要考虑更复杂的情况,比如错误处理、移除监听等。
vue中sessionStorage的使用
在Vue中使用sessionStorage,可以通过以下步骤:
1. 在需要使用sessionStorage的组件中,引入sessionStorage:
```
import sessionStorage from "@/utils/sessionStorage";
```
2. 使用sessionStorage的setItem方法,将数据存储到sessionStorage中:
```
sessionStorage.setItem(key, value);
```
其中,key为存储数据的键名,value为存储的数据。
3. 使用sessionStorage的getItem方法,从sessionStorage中获取数据:
```
sessionStorage.getItem(key);
```
其中,key为要获取数据的键名。
4. 使用sessionStorage的removeItem方法,从sessionStorage中移除数据:
```
sessionStorage.removeItem(key);
```
其中,key为要移除数据的键名。
注意:在使用sessionStorage时,需要注意存储的数据类型,因为sessionStorage只能存储字符串类型的数据。如果需要存储其他类型的数据,可以使用JSON.stringify()将数据转换为字符串类型,使用JSON.parse()将数据转换为对应的类型。
阅读全文
相关推荐
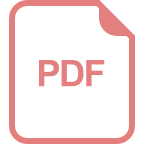
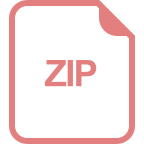
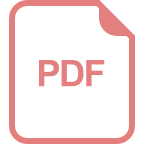

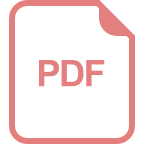
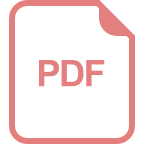
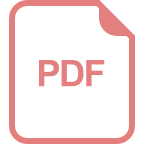
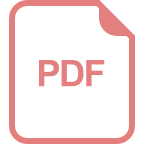
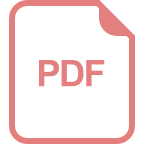
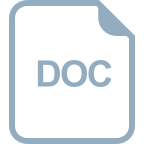
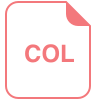




