python编写测试用例
时间: 2023-10-17 17:00:28 浏览: 104
当你在Python中编写测试用例时,可以使用unittest模块或者pytest框架。这两种方法都可以帮助你组织和运行测试。
以下是使用unittest模块编写测试用例的示例:
```python
import unittest
# 要测试的函数或类
def add(a, b):
return a + b
# 编写测试类
class TestAddFunction(unittest.TestCase):
# 每个测试方法以test开头
def test_add_positive_numbers(self):
result = add(3, 5)
self.assertEqual(result, 8)
def test_add_negative_numbers(self):
result = add(-3, -5)
self.assertEqual(result, -8)
def test_add_zero(self):
result = add(3, 0)
self.assertEqual(result, 3)
# 运行测试
if __name__ == '__main__':
unittest.main()
```
在这个示例中,我们编写了一个add函数,并使用unittest模块编写了一个测试类TestAddFunction。测试类中的每个测试方法以`test_`开头,并使用断言方法(例如`assertEqual`)来检查函数的输出是否与预期结果相符。
你可以通过运行`python 文件名.py`来运行测试,unittest模块会自动查找测试类中的所有测试方法并执行。
另外,如果你想使用pytest框架编写测试用例,你需要安装pytest库(`pip install pytest`)。然后,你可以使用类似unittest的方式编写测试用例,或者使用pytest提供的一些简化和增强功能。以下是一个使用pytest编写的类似的示例:
```python
# 要测试的函数或类
def add(a, b):
return a + b
# 使用pytest编写测试用例
def test_add_positive_numbers():
result = add(3, 5)
assert result == 8
def test_add_negative_numbers():
result = add(-3, -5)
assert result == -8
阅读全文
相关推荐
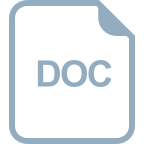
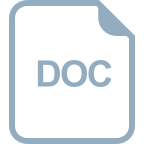
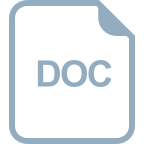
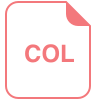








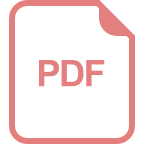
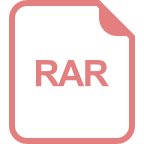
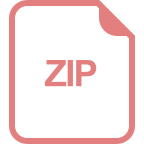
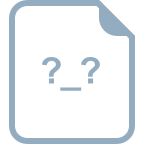
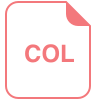

