如何构建函数让数组排序
时间: 2024-10-07 17:00:52 浏览: 28
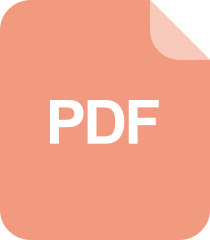
PHP数组排序函数合集 以及它们之间的联系分析
在C++中,你可以使用标准库中的`std::sort`函数对数组进行排序。这是一个例子,展示了如何定义一个函数来对整数类型的数组进行升序排列:
```cpp
#include <algorithm> // 包含sort函数
// 定义一个模板函数,用于对任意类型数组进行排序
template<typename T>
void sortArray(T arr[], int size) {
std::sort(arr, arr + size); // 使用std::sort对数组进行排序
}
int main() {
int numbers[] = {5, 2, 8, 1, 9};
int size = sizeof(numbers) / sizeof(numbers[0]); // 计算数组大小
sortArray(numbers, size);
// 打印排序后的数组
for (int i = 0; i < size; ++i) {
std::cout << numbers[i] << " ";
}
return 0;
}
```
在这个示例中,`sortArray`函数接受一个数组和它的大小作为参数,然后调用`std::sort`函数对整个范围内的元素进行排序。
如果你想自定义排序规则(比如按字符串长度或自定义比较器),你可以提供一个`std::function`类型的比较函数给`std::sort`:
```cpp
bool compareStrings(const std::string& a, const std::string& b) {
return a.length() < b.length(); // 按字符串长度升序
}
// 自定义排序版本
template<typename T>
void customSortArray(T arr[], int size, bool (*compare)(const T&, const T&)) {
std::sort(arr, arr + size, compare);
}
int main() {
std::string words[] = {"banana", "apple", "cherry", "date"};
int wordSize = sizeof(words) / sizeof(words[0]);
customSortArray(words, wordSize, compareStrings);
// ...
}
```
阅读全文
相关推荐
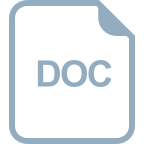
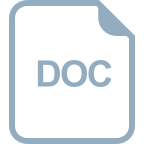
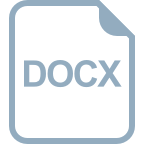
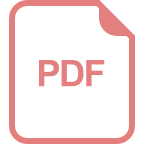
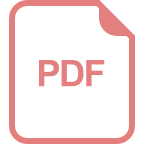
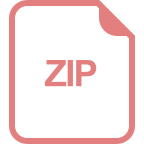
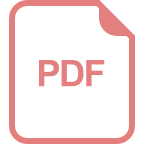
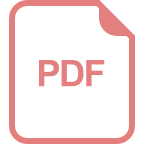
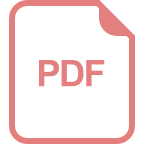
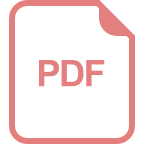
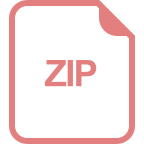
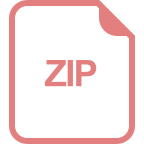
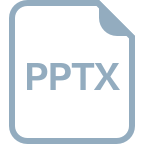
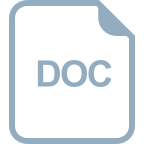