如何在找到的轮廓中绘制轮廓上下底边中点
时间: 2024-10-10 09:15:56 浏览: 22
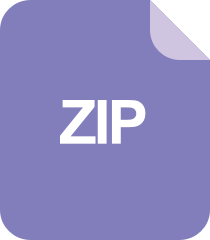
TrueType字体轮廓矢量绘制
在OpenCV中,要从找到的轮廓中绘制轮廓的上边界、下边界以及其中心点,通常需要进行以下几个步骤:
1. **定位轮廓顶点和底部点**:
首先,你需要遍历轮廓的边界点(`approxPolyDP()`函数返回的简化版本)。对于矩形或类似形状的轮廓,顶点通常是水平方向的,可以找到最左边和最右边的点作为上边界;底部点则对应最下面的两个点。
```python
for contour in contours:
x, y, w, h = cv2.boundingRect(contour) # 获取最小包围矩形,x,y为左上角坐标,w,h为宽高
top_left = (x, y) # 上边界左上角
top_right = (x+w, y) # 上边界右上角
bottom_left = (x, y+h) # 下边界左下角
bottom_right = (x+w, y+h) # 下边界右下角
# 你可以选择其中任意两点作为上边界和下边界,这里取左右边界为例
top_edge = [top_left, top_right]
bottom_edge = [bottom_left, bottom_right]
```
2. **计算中心点**:
中心点是通过将矩形的宽度和高度除以2得到的。如果轮廓不是严格的矩形,可能需要额外处理,比如计算轮廓的质心(几何中心)。
```python
if w > 0 and h > 0:
center_x = int((top_left[0] + top_right[0]) / 2)
center_y = int((top_left[1] + bottom_left[1]) / 2)
center_point = (center_x, center_y)
else:
center_point = None
```
3. **绘制点**:
最后,你可以使用`cv2.circle()`函数在图像上绘制这些点。
```python
img_with_points = cv2.copy(img) # 复制原图
cv2.circle(img_with_points, tuple(top_edge[0]), 2, (0, 0, 255), -1) # 绘制上边界点,红色
cv2.circle(img_with_points, tuple(top_edge[1]), 2, (0, 0, 255), -1) # 绘制下边界点,红色
cv2.circle(img_with_points, center_point, 2, (0, 255, 0), -1) # 绘制中心点,绿色
# 显示结果
cv2.imshow("Image with points", img_with_points)
```
阅读全文
相关推荐
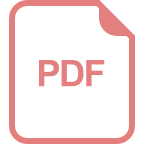
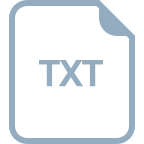
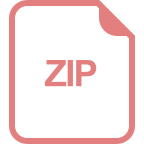
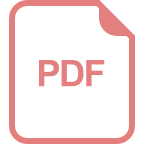
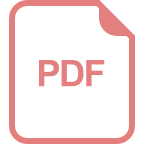
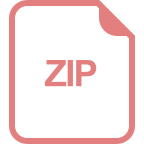
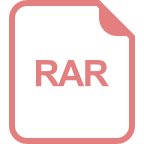
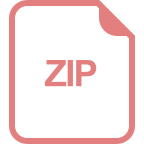
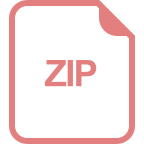
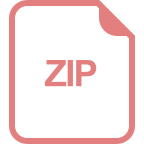
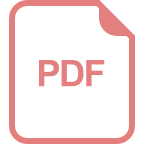
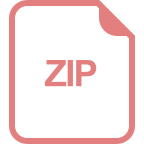
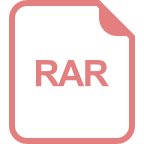
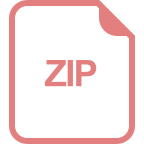
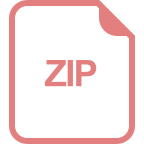
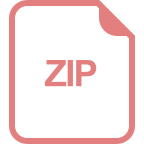