vue中如设置字体颜色
时间: 2024-09-09 16:16:11 浏览: 97
在Vue.js中,设置字体颜色可以通过多种方式实现,主要包括:
1. 内联样式(Inline Styles):可以直接在HTML元素中使用`style`属性来设置样式。例如,要设置一个元素的字体颜色为红色,可以在模板中这样写:
```html
<p :style="{ color: 'red' }">这段文字是红色的。</p>
```
这里使用了`v-bind`(简写为`:`)来绑定一个对象,该对象定义了`color`属性来设置字体颜色。
2. CSS样式文件:可以在组件的`<style>`标签中或者单独的`.css`文件中写入CSS样式规则。例如:
```css
/* 在style标签中或.css文件中 */
.red-text {
color: red;
}
```
然后在Vue模板中应用这个类:
```html
<p class="red-text">这段文字也是红色的。</p>
```
3. JavaScript对象或方法:可以在Vue实例的`data`属性中定义一个对象,该对象包含了样式对象,然后通过`v-bind`将这个样式对象绑定到元素上:
```javascript
new Vue({
el: '#app',
data: {
styleObject: {
color: 'blue'
}
}
});
```
```html
<p :style="styleObject">这段文字是蓝色的。</p>
```
这种方式提供了更多的动态性,可以根据数据的变化来动态改变样式。
相关问题
vue2设置字体颜色
在Vue.js 2中设置字体颜色可以通过以下几种方式实现:
1. 使用内联样式:
在Vue模板中,可以使用`style`属性来设置元素的内联样式,将`color`属性设置为所需的颜色值即可。例如:
```html
<template>
<div style="color: red;">Hello World!</div>
</template>
```
2. 使用类名绑定:
在Vue模板中,可以使用类名绑定来根据数据的状态动态设置元素的类名,然后在CSS中定义相应的样式。例如:
```html
<template>
<div :class="{ 'red-text': isRed }">Hello World!</div>
</template>
<style>
.red-text {
color: red;
}
</style>
```
3. 使用计算属性:
在Vue组件中,可以使用计算属性来根据数据的状态动态计算出所需的样式。例如:
```html
<template>
<div :style="{ color: textColor }">Hello World!</div>
</template>
<script>
export default {
data() {
return {
isRed: true
};
},
computed: {
textColor() {
return this.isRed ? 'red' : 'black';
}
}
};
</script>
```
以上是几种常见的在Vue.js 2中设置字体颜色的方法,你可以根据具体的需求选择适合的方式来实现。
vue3设置字体颜色
在Vue 3中设置字体颜色可以通过以下几种方式实现:
1. 使用内联样式:可以直接在模板中使用内联样式来设置字体颜色。例如:
```html
<template>
<div style="color: red;">Hello Vue 3!</div>
</template>
```
2. 使用类绑定:可以通过绑定一个类来设置字体颜色。首先,在组件的样式中定义一个类,然后使用动态绑定将该类应用到需要设置字体颜色的元素上。例如:
```html
<template>
<div :class="{ 'red-text': isRed }">Hello Vue 3!</div>
</template>
<style>
.red-text {
color: red;
}
</style>
<script>
export default {
data() {
return {
isRed: true
};
}
};
</script>
```
3. 使用计算属性:可以通过计算属性来动态计算字体颜色,并将其应用到元素上。例如:
```html
<template>
<div :style="{ color: textColor }">Hello Vue 3!</div>
</template>
<script>
export default {
computed: {
textColor() {
// 根据条件返回不同的颜色值
return this.isRed ? 'red' : 'blue';
}
}
};
</script>
```
这些是在Vue 3中设置字体颜色的几种常见方式。你可以根据具体的需求选择适合的方法来实现。如果你有其他相关问题,请继续提问。
阅读全文
相关推荐
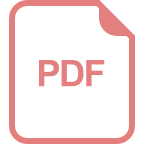
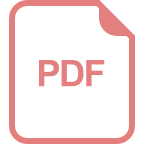
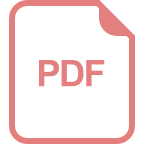













