qtcombobox中的信息如何在另一个标签中显示
时间: 2024-10-20 17:04:43 浏览: 57
在Qt中,`QComboBox`通常用于提供下拉选项让用户选择。如果你想要将用户在`QComboBox`中选择的信息显示到另一个标签(`QLabel`)中,你可以按照以下步骤操作:
1. 创建`QComboBox`实例并添加选项:
```cpp
QComboBox comboBox;
comboBox.addItem("选项1");
comboBox.addItem("选项2");
// 更多选项...
```
2. 将`QComboBox`连接到信号槽系统,当用户选择一个项时,会触发`currentIndexChanged`信号:
```cpp
QObject::connect(&comboBox, &QComboBox::currentIndexChanged, [this](int index) {
// 当选中项发生变化时,获取当前索引对应的值
QString selectedItem = comboBox.itemText(index);
});
```
3. 使用这个`selectedItem`变量设置`QLabel`的内容:
```cpp
QLabel *label = new QLabel(this); // 创建QLabel实例
label->setText(selectedItem); // 更新标签文本
```
4. 如果你想在`QComboBox`的变化实时更新标签,记得将上述代码放在`槽函数`或者`QObject`的生命周期内。
相关问题
qt combobox设置居中显示
在Qt中,如果你想要让QComboBox(组合框)的选项标签或下拉列表部分居中显示,你可以通过调整样式表(Qt Style Sheets)来实现。QComboBox有一个内置的样式属性`QStyle::OptionComboBox`,可以用来控制其外观。
首先,你需要创建一个`.qss`文件或者直接在你的UI中编写样式规则。以下是设置QComboBox垂直居中的基本步骤:
1. 打开你的`mainwindow.ui`或对应的UI文件,并找到`<your_combobox>`的ID(假设它是`comboBox1`),添加`QT_STYLE_SHEET`属性:
```xml
<combobox
...
styleSheet="/* 在这里添加样式 */"
...
objectName="comboBox1">
</combobox>
```
2. 编辑样式表,设置垂直对齐中心。例如:
```css
#comboBox1 {
padding-top: 0px; /* 如果默认有上边距,需要移除 */
height: your_fixed_height; /* 设置固定高度 */
align-items: center; /* 垂直居中 */
min-width: your_min_width;
}
```
请注意替换`your_fixed_height`和`your_min_width`为实际的宽度和高度值。
3. 如果你想让下拉箭头也居中,可以调整`QComboBox::down-arrow`的样式,使其位于底部中央。例如:
```css
QComboBox::down-arrow {
image: url(path_to_your_arrow_icon); /* 路径指向你的下拉箭头图标 */
top: 50%; /* 上半部分与选中的文字对齐 */
left: 50%;
transform: translate(-50%, -50%);
}
```
保存并应用这个样式,你的QComboBox就应该水平居中并且下拉箭头也居中了。
qtdesigner 里comboBox里的项显示不全,增加一个鼠标悬停标签,怎么做
您可以通过使用QComboBox的QAbstractItemView子类来实现此功能。具体步骤如下:
1. 创建一个新类HoverDelegate,继承自QStyledItemDelegate类。
2. 在HoverDelegate类中实现createEditor()和paint()函数,用于创建和绘制悬停标签。
3. 在QComboBox中设置itemDelegate属性为HoverDelegate的实例。
以下是代码示例:
```python
from PyQt5.QtWidgets import QComboBox, QStyledItemDelegate, QLabel, QApplication
from PyQt5.QtGui import QPainter, QColor, QPalette
from PyQt5.QtCore import Qt, QRect
class HoverDelegate(QStyledItemDelegate):
def createEditor(self, parent, option, index):
label = QLabel(parent)
label.setAutoFillBackground(True)
palette = QPalette()
palette.setColor(label.backgroundRole(), QColor(255, 255, 255, 200))
label.setPalette(palette)
label.setAlignment(Qt.AlignCenter)
label.setMargin(2)
label.setWordWrap(True)
label.setText(index.data())
return label
def paint(self, painter, option, index):
if option.state & QStyle.State_MouseOver:
painter.fillRect(option.rect, QColor(200, 200, 200, 100))
painter.drawText(option.rect, Qt.AlignCenter, index.data())
class ComboBox(QComboBox):
def __init__(self, parent=None):
super().__init__(parent)
self.setItemDelegate(HoverDelegate(self))
if __name__ == '__main__':
app = QApplication([])
combo = ComboBox()
combo.addItems(['Item 1', 'Item 2', 'Item 3', 'Item 4', 'Item 5', 'Item 6', 'Item 7', 'Item 8', 'Item 9'])
combo.show()
app.exec_()
```
在这个示例中,我们创建了一个名为HoverDelegate的新类,它继承自QStyledItemDelegate,并重写了createEditor()和paint()函数。createEditor()函数用于创建一个新的QLabel实例作为悬停标签,并设置其样式和文本。paint()函数用于绘制列表项和悬停标签,当鼠标悬停在列表项上时,绘制一个半透明的矩形作为悬停标签。
在ComboBox类的构造函数中,我们将itemDelegate属性设置为HoverDelegate的实例。这将确保在下拉列表中显示项时,使用我们自定义的委托来绘制每个项。
注意:为了使悬停标签覆盖下拉框中的其他项,我们需要将QComboBox的viewMode属性设置为QComboBox.Popup,这将使下拉框显示为一个浮动窗口,而不是下拉列表。
阅读全文
相关推荐
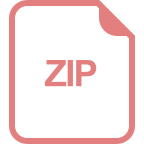
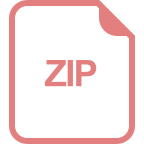
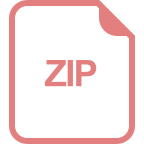
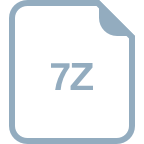
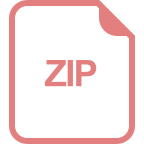
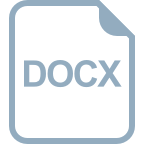
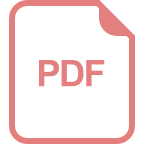
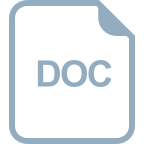
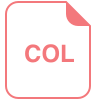
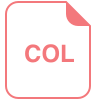







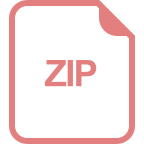