某班有10名学生参加全国计算机等级考试,编写一个程序求该班学生考试成绩的平均分和高于平均分的人数用C++
时间: 2024-11-12 20:39:33 浏览: 41
首先,我们需要创建一个C++程序来计算班级学生的总分数、平均分,并确定有多少人得分高于平均分。这是一个简单的步骤示例:
```cpp
#include <iostream>
#include <vector>
double calculateAverage(const std::vector<int>& scores) {
double sum = 0;
for (int score : scores) {
sum += score;
}
return sum / scores.size();
}
int countAboveAverage(const std::vector<int>& scores, const double& average) {
int count = 0;
for (int score : scores) {
if (score > average) {
count++;
}
}
return count;
}
int main() {
// 假设我们有一个包含10个学生成绩的数组
std::vector<int> studentScores = {85, 90, 78, 92, 88, 86, 94, 89, 91, 87};
// 计算平均分
double totalScore = 0;
for (auto score : studentScores) {
totalScore += score;
}
double averageScore = totalScore / 10;
// 输出平均分
std::cout << "平均分: " << averageScore << std::endl;
// 计算并输出高于平均分的人数
int highScorers = countAboveAverage(studentScores, averageScore);
std::cout << "高于平均分的人数: " << highScorers << std::endl;
return 0;
}
```
在这个程序中,`calculateAverage`函数用于计算平均分,`countAboveAverage`函数则统计高于平均分的学生人数。在`main`函数中,我们模拟了10个学生的成绩,并调用这两个函数。
阅读全文
相关推荐
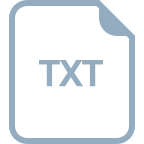

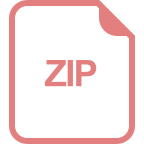
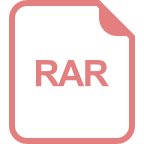
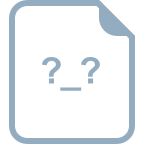
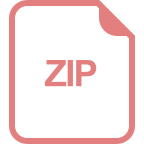
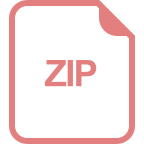
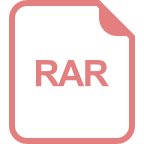
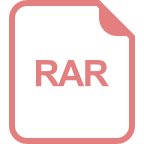
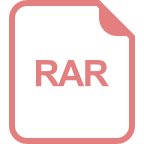
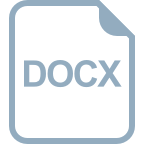