stm32使用mbedtls实现https
时间: 2024-09-06 22:07:16 浏览: 39
STM32微控制器使用Mbed TLS (以前称为 mbedtls) 来支持HTTPS协议,这是一种安全通信协议,基于TCP/IP,用于在客户端(如智能手机、嵌入式设备)和服务器之间传输数据,特别是在互联网上进行敏感信息交换。
以下是使用Mbed TLS在STM32上实现HTTPS的基本步骤:
1. **库配置**:首先,需要将Mbed TLS库添加到你的STM32项目中,并进行适当的初始化,包括配置加密算法、哈希函数等。
```c
#include "mbedtls/config.h"
// ...其他头文件
mbedtls_config config;
mbedtls_ssl_context ssl;
```
2. **证书管理**:你需要准备公钥证书(.pem格式)以及私钥(.key格式),并使用Mbed TLS提供的函数加载它们。
```c
const char *cert_pem = "path_to_your_certificate.pem";
const char *private_key_pem = "path_to_your_private_key.pem";
mbedtls_pk_context pk;
if (mbedtls_pk_parse_key(&pk, private_key_pem, NULL, NULL) != 0) {
// 处理错误
}
mbedtls_x509_crt cacert;
if (mbedtls_x509_crt_parse(&cacert, cert_pem) != 0) {
// 处理错误
}
```
3. **SSL套接字初始化**:创建一个SSL上下文,并设置服务器地址和其他参数。
```c
if (mbedtls_ssl_init(&ssl) != 0) {
// 处理错误
}
if (mbedtls_ssl_setup(&ssl, &config) != 0) {
// 处理错误
}
ssl.server_name = (uint8_t *)"your_server.example.com"; // 设置服务器域名
```
4. **连接建立**:尝试连接到服务器,通过`mbedtls_ssl_connect()`函数开始TLS握手过程。
```c
if (mbedtls_ssl_handshake(&ssl) != MBEDTLS_SUCCESS) {
// 处理错误
}
```
5. **数据传输**:一旦连接成功,就可以使用普通的TCP方式进行读写操作,同时保护数据的安全性。
6. **关闭连接**:完成数据传输后,记得使用`mbedtls_ssl_close_notify()`和`mbedtls_ssl_free(&ssl)`释放资源。
相关推荐
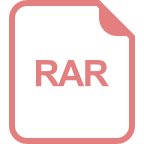
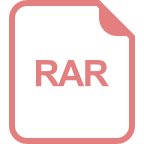
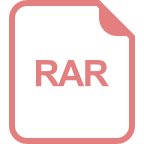














