汉诺塔c语言代码分析
时间: 2024-11-24 08:25:04 浏览: 30
汉诺塔是一个经典的递归问题,它涉及将一组盘子从一根柱子移动到另一根柱子,每次只能移动一个盘子,并且大盘子不能放在小盘子上面。下面是简单的C语言代码实现汉诺塔的递归算法:
```c
#include <stdio.h>
void hanoi(int n, char from_rod, char to_rod, char aux_rod) {
if (n >= 1) {
// Step 1: Move top n - 1 disks from rod A to rod C, using rod B as auxiliary
hanoi(n - 1, from_rod, aux_rod, to_rod);
// Step 2: Move the nth disk from rod A to rod C
printf("Move disk %d from rod %c to rod %c\n", n, from_rod, to_rod);
// Step 3: Move the n - 1 disks that we left on rod C to rod B, using rod A as auxiliary
hanoi(n - 1, aux_rod, to_rod, from_rod);
}
}
int main() {
int num_disks = 3; // You can change this for different number of disks
hanoi(num_disks, 'A', 'C', 'B'); // A, B, and C are the rods in the classic Hanoi problem
return 0;
}
```
这个代码首先定义了一个名为`hanoi`的函数,它接受四个参数:需要移动的盘子数、起始柱子、目标柱子以及辅助柱子。然后通过递归实现三次移动规则(每次只移动一个盘子),直到所有盘子都移动完毕。
阅读全文
相关推荐














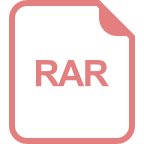



