python筛选出一个文件夹里空的txt文本文件
时间: 2024-10-13 21:08:51 浏览: 39
在Python中,你可以使用`os`和`glob`模块来遍历文件夹并检查哪些`.txt`文件是空的。以下是一个示例代码:
```python
import os
import glob
# 定义要搜索的文件夹路径
folder_path = '/path/to/your/folder'
# 使用glob找出所有的.txt文件
txt_files = glob.glob(os.path.join(folder_path, '*.txt'))
# 空文件判断函数
def is_empty(file_path):
try:
with open(file_path, 'r') as f:
return len(f.read()) == 0
except UnicodeDecodeError:
# 如果文件内容无法解读为文本,通常认为它是空的
return True
# 筛选出空的txt文件
empty_txt_files = [file for file in txt_files if is_empty(file)]
print(f"空的.txt文件有:{empty_txt_files}")
相关问题
python筛选剪切出一个文件夹里空的txt文本文件
在Python中,你可以使用os模块来操作文件和目录,结合shutil库来删除空文件。以下是一个简单的步骤来筛选并剪切(实际这里是删除)文件夹中所有空的.txt文本文件:
```python
import os
import shutil
def filter_empty_txt_files(directory):
# 遍历指定目录及其所有子目录
for root, dirs, files in os.walk(directory):
# 对于每个.txt文件
for file in files:
if file.endswith('.txt'):
# 检查文件大小是否为0,如果是,则它为空
if os.path.getsize(os.path.join(root, file)) == 0:
# 删除(或打印出如果只想检查而不想真的删除)
print(f"Found empty file: {os.path.join(root, file)}")
# 如果你想删除空文件
# shutil.rmtree(os.path.join(root, file), ignore_errors=True)
# 调用函数,并传入你想要检查的文件夹路径
filter_empty_txt_files('/path/to/your/directory')
```
请注意,`shutil.rmtree`会递归地删除整个文件夹,所以在删除前最好确认文件夹中没有其他重要数据。
Python 将同一文件夹下的所有文本文件(.txt文件)合并为一个,合并后的文件名为all.txt
好的,可以使用以下 Python 代码实现:
```python
import os
# 获取当前文件夹下所有的 .txt 文件
txt_files = [f for f in os.listdir() if f.endswith('.txt')]
# 合并所有 .txt 文件内容到 all.txt 文件中
with open('all.txt', 'w', encoding='utf-8') as f:
for txt_file in txt_files:
with open(txt_file, 'r', encoding='utf-8') as tf:
f.write(tf.read())
```
这段代码首先通过 `os.listdir()` 函数获取当前文件夹下所有的文件名,然后筛选出所有以 `.txt` 结尾的文件。接着,使用 `with open()` 语句打开 `all.txt` 文件,并遍历所有 `.txt` 文件,将它们的内容逐一写入到 `all.txt` 文件中。最终得到一个名为 `all.txt` 的文件,其中包含了所有 `.txt` 文件的内容。
阅读全文
相关推荐
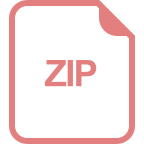
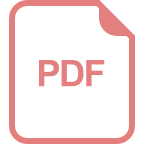
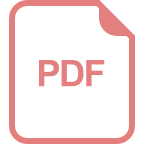
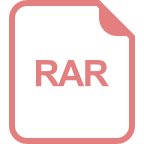
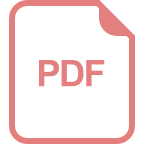
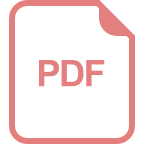
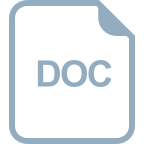
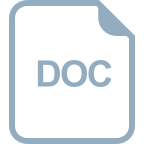
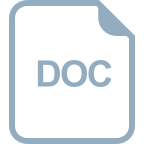







