如何用lstm对上述数据 进行预测,给出python代码,不使用tensorflow和keras
时间: 2024-09-23 12:09:29 浏览: 22
LSTM(长短期记忆网络)是一种循环神经网络,常用于处理序列数据,如时间序列预测。对于预测任务,我们可以直接利用Python的`numpy`库来构建一个简单的LSTM模型。以下是一个基本的示例,展示如何使用`numpy`和`scipy`库来实现LSTM预测,假设我们已经有了预处理的数据(输入X和目标Y):
```python
import numpy as np
from scipy.special import expit # sigmoid函数
# 假设我们有以下数据(形状:(num_samples, time_steps, input_dim))
input_data = ... # (X)
target_data = ... # (Y)
# 定义超参数
input_dim = input_data.shape[-1] # 输入维度
hidden_size = 64 # LSTM隐藏层大小
output_dim = target_data.shape[-1] # 输出维度
learning_rate = 0.01
time_steps = input_data.shape[1]
num_epochs = 100
# 初始化权重矩阵
W_ih = np.random.randn(hidden_size, input_dim) * 0.01
W_hh = np.random.randn(hidden_size, hidden_size) * 0.01
b_ih = np.zeros((hidden_size,))
b_hh = np.zeros((hidden_size,))
W_ho = np.random.randn(output_dim, hidden_size) * 0.01
b_ho = np.zeros((output_dim,))
# 定义激活函数(sigmoid在这里用的是expit)
def sigmoid(x):
return expit(x)
# 模型训练函数
def train(input_seq, target_seq):
for _ in range(num_epochs):
h_t = np.zeros((hidden_size,)) # 初始化隐藏状态
c_t = np.zeros((hidden_size,)) # 初始化细胞状态
loss_sum = 0
for i in range(time_steps):
x_t = input_seq[:, i, :]
y_t = target_seq[:, i]
f_t = sigmoid(np.dot(h_t, W_hh) + np.dot(x_t, W_ih) + b_ih)
i_t = sigmoid(np.dot(h_t, W_hi) + np.dot(x_t, W_ix) + b_ix)
o_t = sigmoid(np.dot(h_t, W_ho) + np.dot(x_t, W_io) + b_io)
c_t_ = f_t * c_t + i_t * np.tanh(np.dot(h_t, W_hc) + np.dot(x_t, W_ic) + b_ic)
h_t_ = o_t * np.tanh(c_t_)
pred_y = np.dot(h_t_, W_yo) + b_yo
error = y_t - pred_y
loss_sum += error ** 2
d_pred_y = -2 * error / len(target_seq)
dh_t_ = np.outer(d_pred_y, W_yo)
dh_t = dh_t_ * (1 - h_t_ ** 2) * o_t
db_yo += d_pred_y
dW_yo += np.outer(h_t_, d_pred_y)
dh_t__ = dh_t * np.tanh(c_t_) * (1 - f_t)
dc_t__ = dh_t__ * i_t
dh_t = dh_t__ * f_t + dh_t * np.dot(W_hh.T, dh_t__)
dW_ho += np.outer(h_t, dh_t)
dW_hc += np.outer(h_t, dh_t_) * np.tanh(c_t__)
dh_t__ *= np.tanh(c_t__)
di_t__ = dh_t__ * np.dot(W_hi.T, dh_t__)
dc_t__ -= di_t__ * o_t
dW_hi += np.outer(h_t, di_t__)
dW_hx += np.outer(x_t, di_t__)
dh_t__ *= f_t
df_t__ = dh_t__ * np.dot(W_hh.T, dh_t__)
dc_t__ -= df_t__ * c_t
dW_hh += np.outer(h_t, df_t__)
dW_ij += np.outer(x_t, df_t__)
c_t = c_t_ + learning_rate * dc_t__
h_t = h_t_ + learning_rate * dh_t__
if i % 10 == 0:
print(f"Epoch {i}: Loss: {loss_sum / len(target_seq)}")
# 使用训练好的模型进行预测
def predict(input_seq):
h_t = np.zeros((hidden_size,))
c_t = np.zeros((hidden_size,))
output_seq = np.zeros((output_dim, time_steps))
for i in range(time_steps):
x_t = input_seq[:, i, :]
f_t, i_t, o_t, c_t_, h_t_ = compute_next_state(x_t, h_t, c_t)
h_t = h_t_
output_seq[:, i] = np.dot(h_t_, W_yo) + b_yo
return output_seq
# 剩余部分是计算下一个时间步的状态,你可以自定义这个函数
def compute_next_state(x_t, h_t, c_t):
...
```
请注意,这只是一个简化的示例,实际应用中你可能需要更复杂的架构,并结合其他优化策略和技巧,例如批量处理、学习率调整等。此外,由于没有使用`scipy.optimize`或其他优化工具,手动更新权重可能会非常耗时并且不太实用。
相关推荐
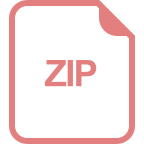
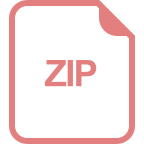
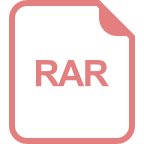














