C知道 有一个3×4的矩阵,要求编程序求出其中值最大的那个元素的值,以及其所在的行号和列号用c语言编写
时间: 2024-12-21 11:23:20 浏览: 12
在C语言中,你可以使用一个简单的遍历过程来寻找3×4矩阵中最大元素及其位置。下面是一个示例程序:
```c
#include <stdio.h>
// 定义矩阵结构体
typedef struct {
int row;
int col;
int value;
} MatrixCell;
// 比较函数,用于找到最大值
int compare(const void *a, const void *b) {
return ((MatrixCell *)a)->value - ((MatrixCell *)b)->value;
}
void findMaxAndCoord(int matrix[3][4], int rows, int cols) {
// 初始化最大值、位置
MatrixCell maxCell = {0, 0, INT_MIN};
// 使用qsort对矩阵按照值进行降序排序
qsort(matrix, rows, sizeof(MatrixCell), compare);
// 最大值的位置就是排序后的第一个元素
maxCell.row = 0;
maxCell.col = (matrix[0].col + 3) % 4; // 因为数组索引从0开始
printf("最大值: %d\n", maxCell.value);
printf("行号: %d\n", maxCell.row + 1); // 加1是因为数组索引是1-based
printf("列号: %d\n", maxCell.col + 1); // 同理
}
int main() {
int matrix[3][4] = {
{5, 2, 9, 1},
{3, 8, 4, 7},
{6, 1, 2, 5}
};
int rows = 3, cols = 4;
findMaxAndCoord(matrix, rows, cols);
return 0;
}
```
这个程序首先创建了一个`MatrixCell`结构体来存储每个矩阵元素及其位置,然后通过`qsort`函数对矩阵按值降序排序。因为二维数组的索引是交错的(如`matrix[row][col]`),所以`col`需要加上3取模4得到对应的列号。
阅读全文
相关推荐
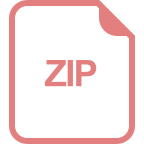
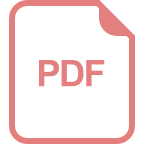
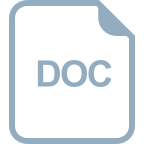















