C++使用boost库通过http异步调用接口并发送json数据的超时处理
时间: 2024-10-27 20:13:26 浏览: 50
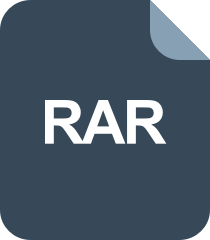
asi-http-request.rar_C++ http 异步_联合开发网讯
在C++中,利用Boost库进行HTTP异步调用接口并发送JSON数据通常会涉及到`asio`库(Asynchronous C++ Socket Interface),它是一个高性能的网络通信库。以下是使用Boost库实现异步HTTP请求,并设置超时处理的一般步骤:
1. **包含必要的库**:
```cpp
#include <boost/beast/core.hpp>
#include <boost/beast/http.hpp>
#include <boost/asio/high_resolution_timer.hpp>
```
2. **创建一个超时处理器**:
```cpp
using net::error_code;
auto timeout_handler = [](error_code ec) {
if (ec == net::errc::operation_timed_out)
std::cerr << "Timeout occurred.\n";
};
```
3. **发起异步请求**:
```cpp
beast::multi_buffer request_body;
// 创建和填充JSON数据到request_body
http::request<http::string_body> req{http::verb::post, "http://example.com/api", version::http/1.1};
req.set(field::content_type, "application/json");
req.body() = beast::buffers_to_string(request_body);
auto resolver = get_io_service().resolve("example.com", 80);
auto strand = io_service::strand {};
auto timer = boost::asio::make_timer(strand.get_executor(), timeout_handler);
// 异步开始连接和发送请求
strand.post([=](asio::yield_context yc) mutable {
tcp::socket socket(io_service);
connect(socket, resolver,yc); // 连接服务器
http::write(socket, req, yc);
strand.wrap(std::move(timer)); // 超时计时器关联到strand上
});
```
4. **异步处理响应**:
```cpp
beast::flat_buffer response_buffer;
http::async_read(socket, response_buffer, beast::bind_front(raw_stream::async_fill_buffer, &response, yc),
strand.wrap([](auto ec) { strand.unwind(); }));
```
5. **设置超时**:
在上述异步读取操作中,`strand.wrap`函数的第二个参数可以用来设置超时时间,比如`timer.expires_from_now(std::chrono::seconds(10))`。
6. **错误检查**:
当异步操作完成时,需要检查错误码判断是否成功,如果超时,错误码将是`net::errc::operation_timed_out`。
注意:以上代码片段展示了一个基本的概念,实际应用中可能需要额外处理解码响应、异常管理以及更复杂的错误路径。
阅读全文
相关推荐
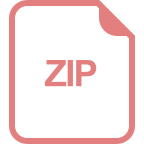
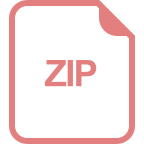
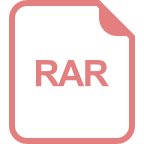
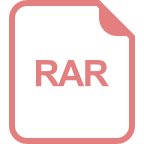
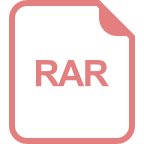
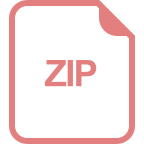
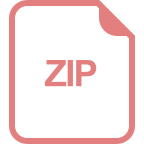
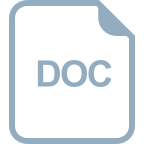
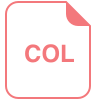
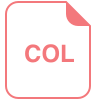
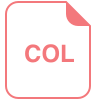
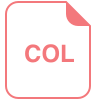

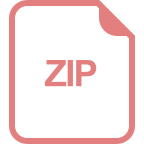
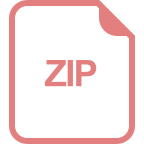