unity 圆角多边形 圆角点怎么创建 C# 实现
时间: 2024-04-19 12:26:16 浏览: 190
要在Unity中创建一个具有圆角点的圆角多边形,你可以使用Unity的内置UI系统和C#编写以下代码:
首先,创建一个空的GameObject并将其命名为"RoundPolygon"。
然后,将Canvas组件添加到该GameObject上,以便在UI中绘制多边形。
接下来,创建一个新的C#脚本,命名为"RoundPolygon.cs",并将其附加到"RoundPolygon" GameObject上。
在脚本中,你可以使用Unity的UI系统和数学函数来绘制圆角多边形。以下是一个例子:
```csharp
using UnityEngine;
using UnityEngine.UI;
public class RoundPolygon : MonoBehaviour
{
public int sides = 6; // 多边形的边数
public float radius = 100f; // 多边形的半径
public float cornerRadius = 10f; // 圆角的半径
void Start()
{
CreateRoundPolygon();
}
void CreateRoundPolygon()
{
// 创建多边形的RectTransform
GameObject polygonObject = new GameObject("Polygon");
polygonObject.transform.SetParent(transform);
RectTransform polygonRectTransform = polygonObject.AddComponent<RectTransform>();
// 添加CanvasRenderer和Image组件以便渲染多边形
CanvasRenderer canvasRenderer = polygonObject.AddComponent<CanvasRenderer>();
Image image = polygonObject.AddComponent<Image>();
image.color = Color.white;
// 创建多边形的顶点数组
Vector2[] polygonVertices = new Vector2[sides];
for (int i = 0; i < sides; i++)
{
float angle = 2 * Mathf.PI / sides * i;
float x = Mathf.Cos(angle) * (radius - cornerRadius) + Mathf.Cos(angle + Mathf.PI / sides) * cornerRadius;
float y = Mathf.Sin(angle) * (radius - cornerRadius) + Mathf.Sin(angle + Mathf.PI / sides) * cornerRadius;
polygonVertices[i] = new Vector2(x, y);
}
// 创建多边形的三角形索引数组
int[] polygonTriangles = new int[3 * (sides - 2)];
for (int i = 0; i < sides - 2; i++)
{
polygonTriangles[3 * i] = 0;
polygonTriangles[3 * i + 1] = i + 1;
polygonTriangles[3 * i + 2] = i + 2;
}
// 设置多边形的顶点和三角形索引
image.sprite = Sprite.Create(Texture2D.whiteTexture, new Rect(0, 0, 1, 1), new Vector2(0.5f, 0.5f));
image.type = Image.Type.Simple;
image.SetNativeSize();
polygonRectTransform.sizeDelta = new Vector2(radius * 2, radius * 2);
image.preserveAspect = true;
image.rectTransform.pivot = new Vector2(0.5f, 0.5f);
image.rectTransform.localPosition = Vector2.zero;
image.rectTransform.localScale = Vector3.one;
image.rectTransform.anchoredPosition = Vector2.zero;
image.rectTransform.anchorMin = new Vector2(0.5f, 0.5f);
image.rectTransform.anchorMax = new Vector2(0.5f, 0.5f);
image.rectTransform.anchoredPosition3D = Vector3.zero;
image.rectTransform.localRotation = Quaternion.identity;
image.rectTransform.sizeDelta = new Vector2(radius * 2, radius * 2);
image.SetVerticesDirty();
image.sprite.OverrideGeometry(polygonVertices, polygonTriangles);
}
}
```
在上面的代码中,我们使用了RectTransform、CanvasRenderer和Image等Unity组件来实现多边形的渲染。通过计算多边形的顶点位置和三角形索引,我们可以绘制出带有圆角点的圆角多边形。
你可以在RoundPolygon脚本中调整sides、radius和cornerRadius的值,以适应你的需求。然后将RoundPolygon脚本附加到RoundPolygon GameObject上,运行游戏即可看到圆角多边形的效果。
希望这能帮到你!
阅读全文
相关推荐
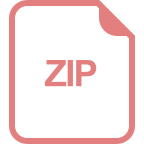
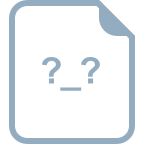
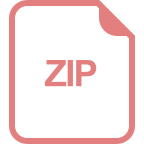
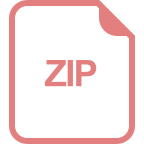
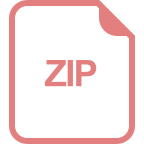
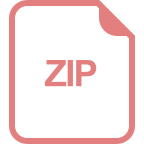
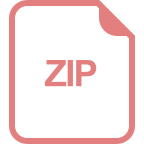
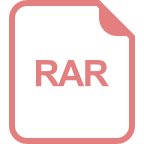
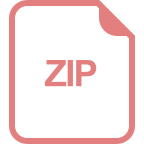
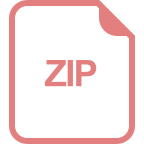
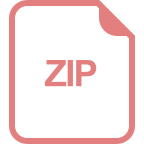
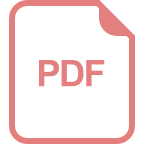
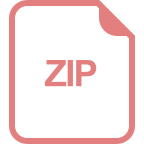