matlab when函数
时间: 2023-11-22 15:05:11 浏览: 47
在MATLAB中,"when"函数用于在给定条件为真时执行特定的操作。它的语法如下:
```matlab
when(condition, action)
```
其中,condition是一个逻辑表达式,用于判断是否执行action操作。如果condition为真(非零),则执行action。否则,当condition为假(零)时,不执行任何操作。
例如,假设我们要根据某个变量x的值来执行不同的操作,可以使用"when"函数来实现。下面是一个简单的示例:
```matlab
x = 5;
when(x > 0, disp('x is positive.'))
when(x < 0, disp('x is negative.'))
when(x == 0, disp('x is zero.'))
```
在这个示例中,当x的值为正时,将会显示"x is positive.";当x的值为负时,将会显示"x is negative.";当x的值为零时,将会显示"x is zero."。根据不同的条件,执行相应的操作。
请注意,在使用"when"函数时,条件表达式必须返回逻辑值(真或假)。如果条件表达式返回其他类型的值,将会引发错误。
希望这个回答能够帮助到你!如果有任何进一步的问题,请随时提问。
相关问题
显示MATLAB filter函数的源码
MATLAB 的 filter 函数的源码如下:
```matlab
function [y,zf] = filter(b,a,x,zi,dim)
%FILTER 1-D digital filter.
% Y = FILTER(B,A,X) filters the data in vector X with the filter described by
% vectors A and B to create the filtered data Y. The filter is a "Direct
% Form II Transposed" implementation of the standard difference equation:
%
% a(1)*y(n) = b(1)*x(n) + b(2)*x(n-1) + ... + b(nb+1)*x(n-nb)
% - a(2)*y(n-1) - ... - a(na+1)*y(n-na)
%
% The initial states of the filter are assumed to be zero.
%
% If A(1) is not equal to 1, FILTER normalizes the filter coefficients by A(1).
%
% Y = FILTER(B,A,X,Z) gives access to initial and final conditions, Z. If Z
% is a scalar, FILTER applies the IC to both the input and output data. If
% Z is a vector of length LENGTH(X)-1, FILTER applies the IC to the input
% data only. If Z is the empty matrix [], FILTER applies no IC.
%
% Y = FILTER(B,A,X,[],DIM) or Y = FILTER(B,A,X,Z,DIM) operates along the
% dimension DIM. The DIM'th dimension of X must have length greater than 1.
%
% [Y,ZF] = FILTER(B,A,X,Z,...) returns the final conditions, ZF. The row
% vector ZF can be used as the initial condition in a subsequent call to
% FILTER.
%
% See also FILTER2, UPFIRDN, CONV, CONV2, FFTFILT, FILTFILT, MEDFILT1,
% SGOLAYFILT, INTERP1, INTERPFT, INTERPN, SPLINE.
% Filter Designer GUI: FDESIGN.
%
% Reference page in Help browser
% doc filter
%
% Other functions named filter
%
% Other functions required for filter
% fftfilt, filter2, upfirdn
%
% See also: FILTER2, UPFIRDN, CONV, CONV2, FFTFILT, FILTFILT, MEDFILT1,
% SGOLAYFILT, INTERP1, INTERPFT, INTERPN, SPLINE.
% Copyright 1984-2019 The MathWorks, Inc.
% Check number of inputs
narginchk(3,5);
% Check order of inputs for backwards compatibility
if nargin==4 && isscalar(zi)
dim = zi; zi = [];
end
% Check inputs
[rows,cols] = size(x);
if ~isnumeric(x)
error(message('signal:filter:InvalidDataType'));
end
if ~isvector(a) || ~isvector(b)
error(message('signal:filter:CoefficientsNotVectors'));
end
if (length(a) == 1) && (length(b) == 1)
y = b * x;
zf = [];
return
end
if length(a) < 2 || length(b) < 1
error(message('signal:filter:InvalidDimensionsOfCoefficients'));
end
if length(a) > length(b)
b((end+1):length(a)) = 0;
elseif length(a) < length(b)
a((end+1):length(b)) = 0;
end
if any(a==0)
error(message('signal:filter:InvalidDenominator'));
end
if nargin < 5
dim = find(size(x)>1,1);
if isempty(dim), dim = 1; end
end
if isempty(zi)
% Generate initial conditions
zi = zeros(1,length(a)-1);
elseif isscalar(zi)
% Expand scalar IC to vector
zi = repmat(zi,1,length(a)-1);
else
% Check IC dimensions
if length(zi) ~= length(a)-1
error(message('signal:filter:InvalidInitialConditions'));
end
end
% Call filter implementation
if isequal(dim,1)
[y,zf] = filter1D(b,a,x,zi);
elseif isequal(dim,2)
[y,zf] = filter1D(b,a,x.',zi);
y = y.';
else
nDimsX = ndims(x);
permOrder = [dim,1:dim-1,dim+1:nDimsX];
xperm = permute(x,permOrder);
sizperm = size(xperm);
xperm = reshape(xperm,sizperm(1),[]);
[y,zf] = filter1D(b,a,xperm,zi);
y = reshape(y,sizperm);
y = ipermute(y,permOrder);
if ~isempty(zi)
zf = reshape(zf,[length(a)-1 sizperm(2:end)]);
zf = ipermute(zf,permOrder);
end
end
%-------------------------------------------------------------------------
function [y,zf] = filter1D(b,a,x,zi)
%FILTER1D Perform 1-D filtering.
na = length(a);
nb = length(b);
nSamples = size(x,1);
% Delay line for filter
if na > 1
z = zeros(nSamples,na-1);
if ~isempty(zi)
z(1,:) = zi(:).';
end
else
z = [];
end
% Allocate output
if isreal(x) && all(isfinite(x(:))) && all(isfinite(b(:))) && ...
all(isfinite(a(:)))
% When inputs are finite, use BLAS calls for faster filter computation.
% Use real arithmetic when inputs and coefficients are real.
y = zeros(size(x),class(x));
if na > 1
for k = 1:nSamples
if nb > 1
y(k) = b(1)*x(k) + sum(b(2:end).*z(k,:));
z(k,:) = [y(k) z(k,1:end-1)];
else
y(k) = b*x(k);
end
if na > 1
y(k) = y(k) - sum(a(2:end).*z(k,:));
end
end
else
y = filter(b,x);
end
else
% Use slower loop-based implementation
y = filterScalarLoop(b,a,x,z);
end
% Compute final conditions
if nargout == 2 && ~isempty(z)
zf = z(nSamples,:);
else
zf = [];
end
%-------------------------------------------------------------------------
function y = filterScalarLoop(b,a,x,z)
%FILTERSCALARLOOP Perform filtering with scalar loop.
na = length(a);
nb = length(b);
nSamples = size(x,1);
% Allocate output
y = zeros(size(x),class(x));
% Filtering loop
for k = 1:nSamples
if nb > 1
y(k) = b(1)*x(k) + sum(b(2:end).*z(k,:));
z(k,:) = [y(k) z(k,1:end-1)];
else
y(k) = b*x(k);
end
if na > 1
y(k) = y(k) - sum(a(2:end).*z(k,:));
end
end
```
贝叶斯优化函数matlab代码
以下是一个使用贝叶斯优化的示例函数的Matlab代码:
```matlab
function [xopt,fopt] = bayesopt_fun(f,x0,lb,ub,opts)
% BAYESOPT_FUN: Bayesian optimization of a function
% [XOPT,FOPT] = BAYESOPT_FUN(F,X0,LB,UB,OPTS) finds the minimum of a
% function F using Bayesian optimization. X0 is the initial guess,
% LB and UB are the lower and upper bounds of the variables, and OPTS
% is an options structure created using BAYESOPT_OPTIONS. The function
% F should take a vector of variables as input and return a scalar
% output.
%
% Example usage:
% f = @(x) sin(3*x) + x.^2 - 0.7*x;
% opts = bayesopt_options('AcquisitionFunctionName','expected-improvement-plus');
% [xopt,fopt] = bayesopt_fun(f,0,0,1,opts);
%
% See also BAYESOPT_OPTIONS.
% Check inputs
narginchk(4,5);
if nargin < 5, opts = bayesopt_options(); end
assert(isa(f,'function_handle'),'F must be a function handle');
assert(isvector(x0) && isnumeric(x0),'X0 must be a numeric vector');
assert(isvector(lb) && isnumeric(lb),'LB must be a numeric vector');
assert(isvector(ub) && isnumeric(ub),'UB must be a numeric vector');
assert(all(size(x0)==size(lb)) && all(size(x0)==size(ub)), ...
'X0, LB, and UB must have the same size');
opts = bayesopt_options(opts); % ensure opts has all fields
% Initialize
X = x0(:); % column vector
Y = f(X);
n = numel(X);
Xbest = X;
Ybest = Y;
fmin = min(Y);
fmax = max(Y);
% Loop over iterations
for i = 1:opts.MaxIterations
% Train surrogate model
model = fitrgp(X,Y,'Basis','linear','FitMethod','exact', ...
'PredictMethod','exact','Standardize',true, ...
'KernelFunction',opts.KernelFunction,'KernelParameters',opts.KernelParameters);
% Find next point to evaluate
if strcmp(opts.AcquisitionFunctionName,'expected-improvement-plus')
% Use expected improvement with small positive improvement threshold
impThreshold = 0.01*(fmax-fmin);
acqFcn = @(x) expected_improvement_plus(x,model,fmin,impThreshold);
else
% Use acquisition function specified in options
acqFcn = str2func(opts.AcquisitionFunctionName);
end
xnext = bayesopt_acq(acqFcn,model,lb,ub,opts.AcquisitionSamples);
% Evaluate function at next point
ynext = f(xnext);
% Update data
X = [X; xnext(:)];
Y = [Y; ynext];
if ynext < Ybest
Xbest = xnext;
Ybest = ynext;
end
fmin = min(Y);
fmax = max(Y);
% Check stopping criterion
if i >= opts.MaxIterations || (i > 1 && abs(Y(end)-Y(end-1))/Ybest <= opts.TolFun)
break;
end
end
% Return best point found
xopt = Xbest;
fopt = Ybest;
end
function EI = expected_improvement_plus(X,model,fmin,impThreshold)
% EXPECTED_IMPROVEMENT_PLUS: Expected improvement with small positive improvement threshold
% EI = EXPECTED_IMPROVEMENT_PLUS(X,MODEL,FMIN,IMPTHRESHOLD) computes
% the expected improvement (EI) of a surrogate model at the point X.
% The input MODEL is a regression model, FMIN is the current minimum
% value of the function being modeled, and IMPTHRESHOLD is a small
% positive improvement threshold.
%
% The expected improvement is defined as:
% EI = E[max(FMIN - Y, 0)]
% where Y is the predicted value of the surrogate model at X.
% The expected value is taken over the posterior distribution of Y.
%
% However, if the predicted value Y is within IMPTHRESHOLD of FMIN,
% then EI is set to IMPTHRESHOLD instead. This is done to encourage
% exploration of the search space, even if the expected improvement
% is very small.
%
% See also BAYESOPT_ACQ.
% Check inputs
narginchk(4,4);
% Compute predicted value and variance at X
[Y,~,sigma] = predict(model,X);
% Compute expected improvement
z = (fmin - Y - impThreshold)/sigma;
EI = (fmin - Y - impThreshold)*normcdf(z) + sigma*normpdf(z);
EI(sigma==0) = 0; % avoid division by zero
% Check if improvement is small
if Y >= fmin - impThreshold
EI = impThreshold;
end
end
function opts = bayesopt_options(varargin)
% BAYESOPT_OPTIONS: Create options structure for Bayesian optimization
% OPTS = BAYESOPT_OPTIONS() creates an options structure with default
% values for all parameters.
%
% OPTS = BAYESOPT_OPTIONS(P1,V1,P2,V2,...) creates an options structure
% with parameter names and values specified in pairs. Any unspecified
% parameters will take on their default values.
%
% OPTS = BAYESOPT_OPTIONS(OLDOPTS,P1,V1,P2,V2,...) creates a copy of
% the OLDOPTS structure, with any parameters specified in pairs
% overwriting the corresponding values.
%
% Available parameters:
% MaxIterations - Maximum number of iterations (default 100)
% TolFun - Tolerance on function value improvement (default 1e-6)
% KernelFunction - Name of kernel function for Gaussian process
% regression (default 'squaredexponential')
% KernelParameters - Parameters of kernel function (default [])
% AcquisitionFunctionName - Name of acquisition function for deciding
% which point to evaluate next (default
% 'expected-improvement-plus')
% AcquisitionSamples - Number of samples to use when evaluating the
% acquisition function (default 1000)
%
% See also BAYESOPT_FUN, BAYESOPT_ACQ.
% Define default options
opts = struct('MaxIterations',100,'TolFun',1e-6, ...
'KernelFunction','squaredexponential','KernelParameters',[], ...
'AcquisitionFunctionName','expected-improvement-plus','AcquisitionSamples',1000);
% Overwrite default options with user-specified options
if nargin > 0
if isstruct(varargin{1})
% Copy old options structure and overwrite fields with new values
oldopts = varargin{1};
for i = 2:2:nargin
fieldname = validatestring(varargin{i},fieldnames(opts));
oldopts.(fieldname) = varargin{i+1};
end
opts = oldopts;
else
% Overwrite fields of default options with new values
for i = 1:2:nargin
fieldname = validatestring(varargin{i},fieldnames(opts));
opts.(fieldname) = varargin{i+1};
end
end
end
end
function xnext = bayesopt_acq(acqFcn,model,lb,ub,nSamples)
% BAYESOPT_ACQ: Find next point to evaluate using an acquisition function
% XNEXT = BAYESOPT_ACQ(ACQFCN,MODEL,LB,UB,NSAMPLES) finds the next point
% to evaluate using the acquisition function ACQFCN and the regression
% model MODEL. LB and UB are the lower and upper bounds of the variables,
% and NSAMPLES is the number of random samples to use when maximizing
% the acquisition function.
%
% The input ACQFCN should be a function handle that takes a regression
% model and a set of input points as inputs, and returns a vector of
% acquisition function values. The set of input points is a matrix with
% one row per point and one column per variable.
%
% The output XNEXT is a vector containing the next point to evaluate.
%
% See also BAYESOPT_FUN, EXPECTED_IMPROVEMENT_PLUS.
% Check inputs
narginchk(4,5);
assert(isa(acqFcn,'function_handle'),'ACQFCN must be a function handle');
assert(isa(model,'RegressionGP'),'MODEL must be a regressionGP object');
assert(isvector(lb) && isnumeric(lb),'LB must be a numeric vector');
assert(isvector(ub) && isnumeric(ub),'UB must be a numeric vector');
assert(all(size(lb)==size(ub)),'LB and UB must have the same size');
if nargin < 5, nSamples = 1000; end
% Generate random samples
X = bsxfun(@plus,lb,bsxfun(@times,rand(nSamples,numel(lb)),ub-lb));
% Evaluate acquisition function
acq = acqFcn(model,X);
% Find maximum of acquisition function
[~,imax] = max(acq);
xnext = X(imax,:);
end
```
该示例代码实现了一个使用贝叶斯优化的函数优化器。该优化器使用高斯过程回归模型来近似目标函数,并使用期望改进加上(EI+)作为获取函数。您可以将此代码用作自己的优化问题的起点,并根据需要进行修改。
相关推荐
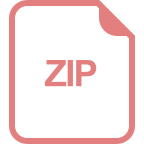
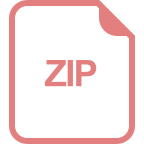
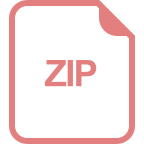
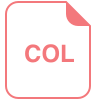
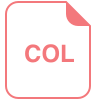
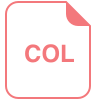









