C#SQLite插入数据库试,报错提示UNIQUE constraint failes:fileFuncExeStatus.CSVPartName
时间: 2024-11-15 19:25:01 浏览: 0
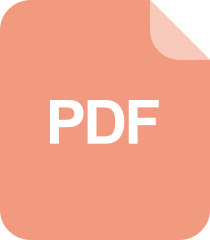
C#/.Net 中快速批量给SQLite数据库插入测试数据
在C#中使用SQLite插入数据时,如果遇到错误提示“UNIQUE constraint failed: fileFuncExeStatus.CSVPartName”,这通常意味着你试图插入的数据违反了数据库中定义的唯一性约束。具体来说,`fileFuncExeStatus`表中的`CSVPartName`列被定义为唯一(UNIQUE),而你尝试插入的值在该列中已经存在。
以下是一些可能的解决方案和检查步骤:
1. **检查现有数据**:
确认你要插入的数据是否已经存在于`fileFuncExeStatus`表的`CSVPartName`列中。你可以运行一个查询来查看当前表中的数据。
```sql
SELECT * FROM fileFuncExeStatus WHERE CSVPartName = 'your_value';
```
2. **修改插入逻辑**:
如果你希望在插入重复值时进行更新而不是插入,可以使用`INSERT OR REPLACE`或`INSERT OR IGNORE`语句。
- `INSERT OR REPLACE`:如果记录已存在,则替换它。
```csharp
string query = "INSERT OR REPLACE INTO fileFuncExeStatus (CSVPartName, other_column) VALUES (@CSVPartName, @other_column)";
```
- `INSERT OR IGNORE`:如果记录已存在,则忽略插入操作。
```csharp
string query = "INSERT OR IGNORE INTO fileFuncExeStatus (CSVPartName, other_column) VALUES (@CSVPartName, @other_column)";
```
3. **处理冲突**:
如果你希望在遇到冲突时执行某些特定操作,比如更新现有记录,可以使用`ON CONFLICT`子句。
```csharp
string query = "INSERT INTO fileFuncExeStatus (CSVPartName, other_column) VALUES (@CSVPartName, @other_column) ON CONFLICT(CSVPartName) DO UPDATE SET other_column = excluded.other_column;";
```
4. **调试代码**:
确保你的插入代码正确无误,并且传递的参数没有误。例如:
```csharp
using (var connection = new SQLiteConnection("Data Source=your_database.db"))
{
connection.Open();
string query = "INSERT INTO fileFuncExeStatus (CSVPartName, other_column) VALUES (@CSVPartName, @other_column)";
using (var command = new SQLiteCommand(query, connection))
{
command.Parameters.AddWithValue("@CSVPartName", yourCSVPartName);
command.Parameters.AddWithValue("@other_column", yourOtherColumnValue);
command.ExecuteNonQuery();
}
}
```
5. **检查表结构**:
确认表结构是否正确,特别是`CSVPartName`列的定义。
```sql
PRAGMA table_info(fileFuncExeStatus);
```
通过以上步骤,你应该能够找到并解决“UNIQUE constraint failed: fileFuncExeStatus.CSVPartName”错误的原因。
阅读全文
相关推荐
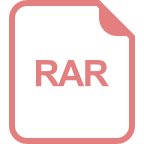
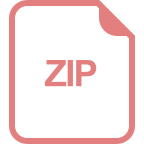
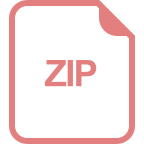
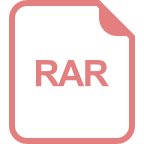
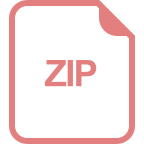
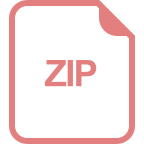
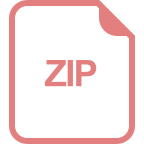
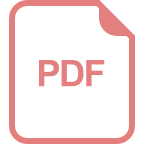
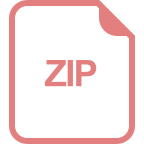
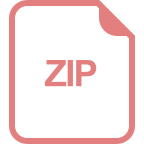
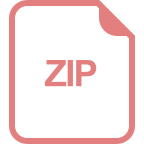
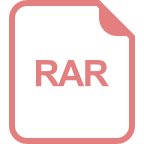
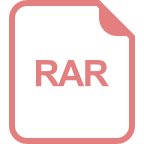
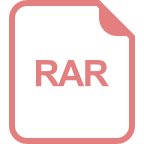
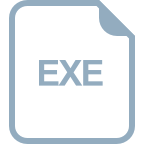
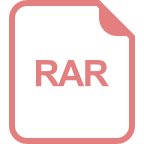
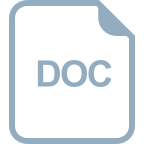
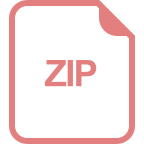