请问可以生成这个文档中的代码运行结果吗
时间: 2024-12-13 15:18:34 浏览: 2
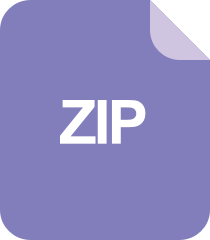
C++代码文档生成器 根据代码及注释自动生成代码文档.zip

根据您提供的文档内容,以下是各个部分的预期代码运行结果和解释:
### 1. 一程序两进程
**代码:**
```c
#include <stdio.h>
main() {
int pid, i;
while ((pid = fork()) == -1);
if (pid != 0) {
while (1) {
for (i = 0; i < 100000000; i++);
printf("parent");
}
} else {
while (1) {
for (i = 0; i < 100000000; i++);
printf("child");
}
}
}
```
**运行结果:**
```
parentchildparentchild...
```
**说明:**
- 父进程和子进程同时运行,分别打印 "parent" 和 "child"。
- 由于两个进程是并发执行的,因此输出会交错出现。
### 2. 父子进程之间代码段与数据段的关系
**代码:**
```c
#include <stdio.h>
main() {
int pid, n = 0, i;
while ((pid = fork()) == -1);
if (pid != 0) {
while (1) {
printf("%8d\n", n++);
for (i = 0; i < 100000000; i++);
printf("parent");
}
} else {
while (1) {
printf("%8d\n", n++);
for (i = 0; i < 100000000; i++);
printf("child");
}
}
}
```
**运行结果:**
```
0
parent
1
parent
0
child
1
child
...
```
**说明:**
- 父进程和子进程共享相同的代码段,但各自拥有独立的数据段。
- 因此,变量 `n` 在父进程和子进程中分别计数,导致输出交错且独立增加。
### 3. 两程序两进程
**主程序 (`fork3.c`):**
```c
#include <stdio.h>
main() {
int pid, n = 0, i;
while ((pid = fork()) == -1);
if (pid != 0) {
while (1) {
printf("%8d\n", n++);
for (i = 0; i < 100000000; i++);
printf("parent");
}
} else {
execve("./sp", 0, 0);
}
}
```
**子程序 (`sp.c`):**
```c
main() {
int n = 0, i;
while (1) {
printf("%8d\n", n++);
for (i = 0; i < 100000000; i++);
printf("child");
}
}
```
**运行结果:**
```
0
parent
1
parent
0
child
1
child
...
```
**说明:**
- 主程序创建一个子进程,并使用 `execve` 替换子进程的代码段。
- 父进程继续执行原程序,而子进程执行新的程序。
### 4. 子进程输入十个数,父进程输出这十个数
**代码:**
```c
#include <stdio.h>
main() {
int pid, i, a[10];
while ((pid = fork()) == -1);
if (pid != 0) {
wait(0);
for (i = 0; i < 10; i++)
printf("%4d", a[i]);
} else {
for (i = 0; i < 10; i++)
scanf("%d", &a[i]);
}
}
```
**运行结果:**
```
请输入10个数:
1 2 3 4 5 6 7 8 9 10
1 2 3 4 5 6 7 8 9 10
```
**说明:**
- 子进程先运行,输入10个数。
- 父进程在子进程结束后输出这些数。
### 5. 利用管道实现父子进程合作
**代码:**
```c
#include <stdio.h>
main() {
int pid, i, a[10], fd[2];
pipe(fd);
while ((pid = fork()) == -1);
if (pid != 0) {
wait(0);
read(fd[0], a, 40);
for (i = 0; i < 10; i++)
printf("%4d", a[i]);
} else {
for (i = 0; i < 10; i++)
scanf("%d", &a[i]);
write(fd[1], a, 40);
}
}
```
**运行结果:**
```
请输入10个数:
1 2 3 4 5 6 7 8 9 10
1 2 3 4 5 6 7 8 9 10
```
**说明:**
- 子进程先运行,输入10个数并通过管道传递给父进程。
- 父进程在子进程结束后读取这些数并输出。
### 6. 一程序三进程
**代码:**
```c
#include <stdio.h>
main() {
int pid1, pid2;
while ((pid1 = fork()) == -1);
if (pid1 == 0) {
while ((pid2 = fork()) == -1);
if (pid2 == 0) {
while (1)
printf("process2");
} else {
while (1)
printf("process1");
}
} else {
while (1)
printf("process3");
}
}
```
**运行结果:**
```
process1process2process3process1process2process3...
```
**说明:**
- 第一次 `fork` 创建第一个子进程,第二次 `fork` 在第一个子进程中创建第二个子进程。
- 父进程、第一个子进程和第二个子进程分别打印 "process3"、"process1" 和 "process2"。
### 总结
- **多进程的特点:**
- **创建后特点:** 每个进程都有独立的地址空间,共享代码段,但各自拥有独立的数据段。
- **执行特点:** 进程是并发执行的,操作系统负责调度不同的进程,使得多个进程可以同时运行。
- **多进程编程的结论:**
- 使用 `fork()` 创建子进程,`execve()` 替换进程的代码段,`pipe()` 实现进程间的通信。
- 进程间可以通过信号量、互斥锁等机制进行同步和通信,以保证数据的一致性和正确性。
阅读全文
相关推荐
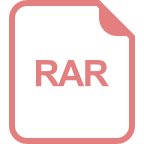
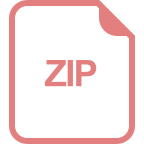
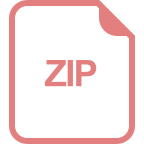
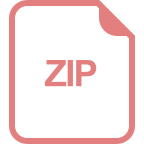
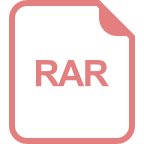
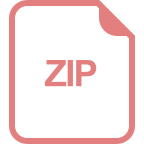
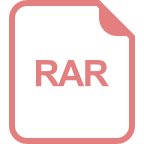
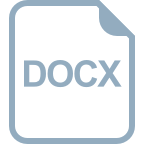
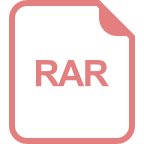
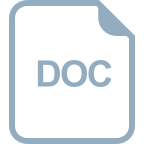
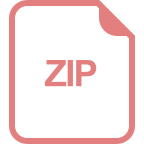
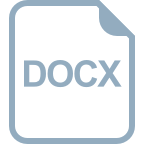
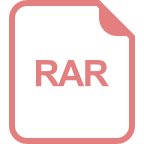
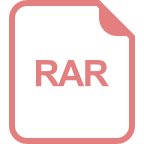
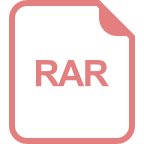
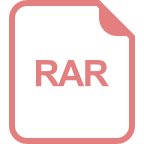
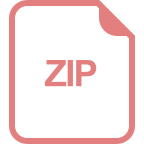
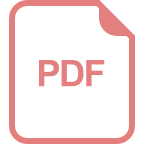