passing argument 1 of 'strlen' makes pointer from integer without a cast [-Wint-conversion]
时间: 2024-09-29 20:08:54 浏览: 172
这个警告是在C/C++编译器中出现的,特别是GCC和Clang这样的工具。"passing argument 1 of 'strlen'"意味着你在调用`strlen`函数时,传递了一个整数类型的参数给它,而`strlen`通常需要一个指向字符数组的指针作为输入。
`strlen`函数用于计算字符串(即字符数组)的长度,所以当你直接提供一个整数值,编译器会发出这个警告,因为它试图将整数转换成指针类型,但没有显式地通过类型转换(cast)。这可能导致未定义的行为,因为整数并不表示内存地址。
为了避免这个警告并确保代码正确,你应该确保传递的是一个有效的字符数组的指针,例如:
```c
char str[] = "Hello, World!";
size_t len = strlen(str); // 正确的方式
```
如果想计算字符串常量的长度,可以忽略警告,因为在C++中,编译器会隐式把字符串字面量转换为指向第一个字符的指针:
```c++
const char* str = "Hello, World!"; // 不需要cast,编译器自动处理
size_t len = strlen(str);
```
相关问题
passing argument 1 of 'output' makes pointer from integer without a cast [-Wint-conversion]
这个警告是在C或C++编程中出现的,当你试图将一个整数类型的变量作为参数传递给一个需要指针类型(如函数指针或指向某个数据结构的指针)的函数或方法时,编译器会发出`[-Wint-conversion]`。警告提示你,从整数到指针的转换没有明确的类型转换(cast),这可能是潜在错误,因为没有指定如何将这个值转化为正确的内存地址。
例如:
```cpp
void output(int* ptr) {
// 函数期望一个整数指针
}
int value = 42;
output(value); // 这里会触发警告,value是一个整数,不是指针
```
为了消除警告并正确地传递,你需要在调用时将整数值转换为指针,比如这样:
```cpp
int value = 42;
output(reinterpret_cast<int*>(&value)); // 使用reinterpret_cast进行类型转换
```
或者如果你确信`value`地址可以安全地作为`int*`处理,可以去掉cast:
```cpp
// 如果value存储在栈上,这样做通常是安全的,但请注意这取决于上下文
output(&value);
```
passing argument 1 of 'search' makes pointer from integer without a cast [-Wint-conversion]
This warning message typically occurs when you are passing an integer value to a function that expects a pointer as its argument. The compiler is warning you that there is a mismatch between the type of the argument you are passing and the type the function expects.
To fix this warning, you should ensure that you are passing the correct type of argument to the function. If the function expects a pointer, you should pass a pointer variable or address. If you are passing an integer value, you may need to cast it to a pointer type before passing it to the function.
For example, consider the following code:
```
int search(int *array, int size, int key) {
// implementation of search function
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int key = 3;
int index = search(key, arr, 5);
// rest of the code
}
```
Here, we are passing the arguments to the `search` function in the wrong order. The function expects an array pointer as its first argument, but we are passing an integer value (`key`) instead. To fix this, we should swap the order of the arguments:
```
int main() {
int arr[] = {1, 2, 3, 4, 5};
int key = 3;
int index = search(arr, 5, key);
// rest of the code
}
```
If you are still getting the warning message after correcting the argument types, you may need to check the function declaration to ensure that it matches the expected argument types.
阅读全文
相关推荐
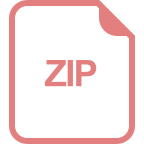
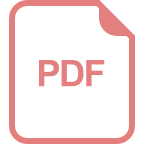














