已知最大速度 运动距离 给出速度规划算法 c语言实现
时间: 2023-12-15 21:36:58 浏览: 127
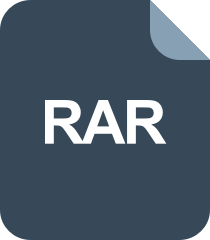
基于目标距离的S型速度曲线规划 C语言实现

在C语言中,可以使用以下速度规划算法来计算速度的变化:
```c
#include <stdio.h>
#include <math.h>
// 速度规划算法
void velocityPlanning(double maxVelocity, double distance, double* velocities, int numPoints) {
double acceleration = pow(maxVelocity, 2) / distance; // 计算加速度
double t_acc = maxVelocity / acceleration; // 加速时间
double t_dec = t_acc; // 减速时间
double t_const = (distance / maxVelocity) - (2 * t_acc); // 匀速时间
double dt = (t_acc + t_const + t_dec) / (numPoints - 1); // 时间间隔
for (int i = 0; i < numPoints; i++) {
double t = i * dt;
if (t <= t_acc) {
velocities[i] = acceleration * t; // 加速阶段
}
else if (t > t_acc && t <= (t_acc + t_const)) {
velocities[i] = maxVelocity; // 匀速阶段
}
else {
double t_dec_offset = t - t_acc - t_const;
velocities[i] = maxVelocity - (acceleration * t_dec_offset); // 减速阶段
}
}
}
int main() {
double maxVelocity = 10.0; // 最大速度
double distance = 100.0; // 运动距离
int numPoints = 100; // 离散点数
double velocities[numPoints]; // 存储速度值的数组
velocityPlanning(maxVelocity, distance, velocities, numPoints);
// 打印速度值
for (int i = 0; i < numPoints; i++) {
printf("Velocity at point %d: %lf\n", i, velocities[i]);
}
return 0;
}
```
在上面的代码中,我们定义了一个 `velocityPlanning` 函数,该函数接受最大速度、运动距离、存储速度值的数组和离散点数作为参数。该函数使用了加速度规划算法来计算速度的变化。
在 `main` 函数中,我们设置了最大速度为10.0,运动距离为100.0。然后,我们调用 `velocityPlanning` 函数来计算速度的变化,并打印出每个离散点的速度值。
请注意,该算法是一个简化的版本,仅用于说明速度规划的基本原理。在实际应用中,可能需要考虑更多因素,如加速度限制、减速时间等。
阅读全文
相关推荐
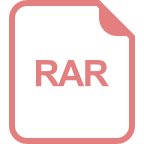
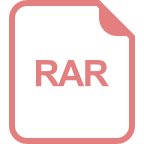
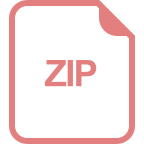
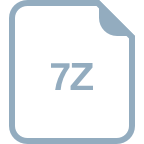
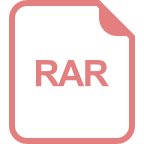
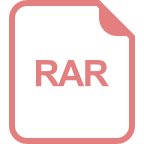
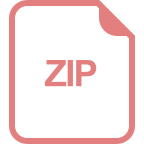
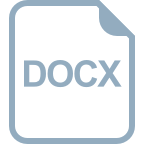
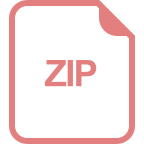
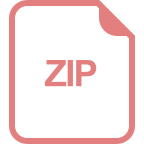
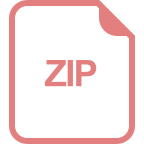
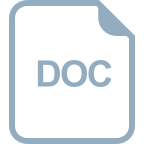
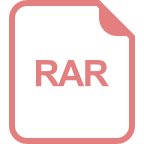
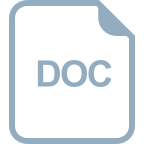
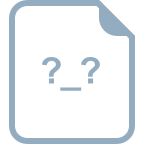