Java 使用Socket进行tcp通信 使用ProtoBuf进行序列化 解码参靠使用Base 128 Varints
时间: 2024-04-19 16:25:08 浏览: 232
你好!关于你的问题,我可以给你一些建议和解释。
Java中使用Socket进行TCP通信是很常见的。你可以使用Java中的Socket类和ServerSocket类来实现TCP客户端和服务器端的通信。通过创建Socket对象,你可以连接到服务器,并通过输入输出流发送和接收数据。
关于使用ProtoBuf进行序列化,ProtoBuf是一种用于结构化数据的高效序列化格式。它可以通过定义消息的结构和字段来生成Java类,并使用这些类进行序列化和反序列化操作。你可以使用Google的protobuf-java库来在Java中使用ProtoBuf。
至于解码参靠使用Base 128 Varints,Base 128 Varints是一种变长整数编码方案,用于节省存储空间。它将整数拆分成多个字节,并使用最高位来表示是否还有更多字节。你可以使用相应的算法来解码Base 128 Varints编码的数据。
希望这些信息对你有帮助!如果你有任何其他问题,请随时提问。
相关问题
Java中 TCP 使用 ProtoBuf 进行序列化请求服务器 返回消息解 码 参 靠 使 用 Base 128 Varints,请写一个请求服务器的示例
当然,我可以为您提供一个使用Java中的TCP和ProtoBuf进行序列化请求服务器并使用Base 128 Varints解码返回消息的示例。以下是一个简单的示例代码:
```java
import com.google.protobuf.ByteString;
import com.google.protobuf.InvalidProtocolBufferException;
import com.google.protobuf.MessageLite;
import com.google.protobuf.Parser;
import java.io.DataInputStream;
import java.io.DataOutputStream;
import java.io.IOException;
import java.net.Socket;
public class TCPClient {
private static final String SERVER_IP = "127.0.0.1";
private static final int SERVER_PORT = 8080;
public static void main(String[] args) {
// 创建请求消息
PersonProto.Person person = PersonProto.Person.newBuilder()
.setId(123)
.setName("John Doe")
.setEmail("johndoe@example.com")
.build();
// 序列化请求消息
byte[] requestBytes = person.toByteArray();
try (Socket socket = new Socket(SERVER_IP, SERVER_PORT)) {
// 发送请求消息
DataOutputStream out = new DataOutputStream(socket.getOutputStream());
out.writeInt(requestBytes.length);
out.write(requestBytes);
out.flush();
// 接收并解码返回消息
DataInputStream in = new DataInputStream(socket.getInputStream());
int responseLength = in.readInt();
byte[] responseBytes = new byte[responseLength];
in.readFully(responseBytes);
PersonProto.Person responsePerson = decodeResponse(responseBytes, PersonProto.Person.parser());
System.out.println("Received response: " + responsePerson);
} catch (IOException e) {
e.printStackTrace();
}
}
private static <T extends MessageLite> T decodeResponse(byte[] responseBytes, Parser<T> parser)
throws InvalidProtocolBufferException {
// 使用Base 128 Varints解码返回消息
ByteString byteString = ByteString.copyFrom(responseBytes);
return parser.parseFrom(byteString);
}
}
```
请确保您已经导入了Google的protobuf库,并且已经定义了您的Person消息类型(示例中使用名为PersonProto的Proto文件)。您可以根据自己的需求进行相应的修改。
如何使用protobuf-net进行序列化和反序列化?
protobuf-net是一个用于序列化和反序列化的库,它是Google Protocol Buffers的一个.NET实现。下面是使用protobuf-net进行序列化和反序列化的步骤:
1. 定义消息结构:首先,你需要定义你的消息结构,可以使用.proto文件来描述消息结构,然后使用protobuf编译器生成对应的C#类。
2. 安装protobuf-net库:在你的项目中安装protobuf-net库,可以通过NuGet包管理器或手动下载安装。
3. 序列化对象:使用protobuf-net库的Serializer.Serialize方法将对象序列化为字节数组。例如:
```csharp
using (var stream = new MemoryStream())
{
Serializer.Serialize(stream, yourObject);
byte[] bytes = stream.ToArray();
}
```
4. 反序列化对象:使用protobuf-net库的Serializer.Deserialize方法将字节数组反序列化为对象。例如:
```csharp
using (var stream = new MemoryStream(bytes))
{
YourObjectType deserializedObject = Serializer.Deserialize<YourObjectType>(stream);
}
```
注意:在进行反序列化时,需要提供目标对象的类型。
阅读全文
相关推荐
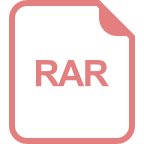
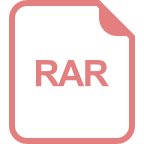
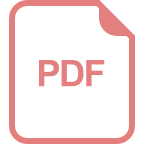













