c语言写一个函数,将两个字符串连接
时间: 2024-12-10 16:51:25 浏览: 26
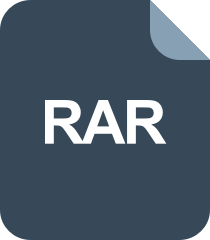
用C语言写一个函数,将两个字符串连接
在C语言中,你可以通过动态内存分配和指针操作来编写一个函数,用于连接两个字符串。下面是一个简单的例子:
```c
#include <stdio.h>
#include <stdlib.h> // 需要包含stdlib头文件以使用malloc()
// 函数声明,返回值为指向新字符串的指针
char* concatStrings(const char* str1, const char* str2);
int main() {
char* result = concatStrings("Hello", " World!");
printf("%s\n", result); // 输出: Hello World!
free(result); // 释放内存
return 0;
}
// 实现concatStrings函数
char* concatStrings(const char* str1, const char* str2) {
int len1 = strlen(str1);
int len2 = strlen(str2);
char* combined = (char*) malloc(len1 + len2 + 1); // +1是为了放终止符'\0'
if(combined == NULL) { // 检查内存分配是否成功
printf("Memory allocation failed.\n");
return NULL;
}
strcpy(combined, str1); // 将str1复制到combined
strcat(combined, str2); // 连接str2到combined
return combined; // 返回新的字符串
}
```
这个`concatStrings`函数首先计算两个输入字符串的总长度,然后动态分配足够的内存存储它们以及一个额外的空间存放终止符。接着它将第一个字符串复制过去,并使用`strcat`函数添加第二个字符串。最后返回连接后的字符串,但在main函数结束后别忘了释放之前动态分配的内存。
阅读全文
相关推荐
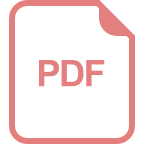
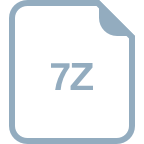
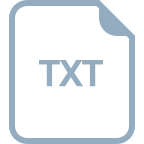
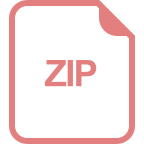
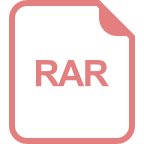
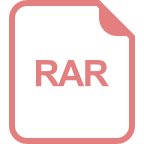
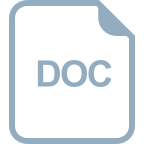
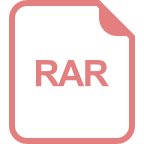
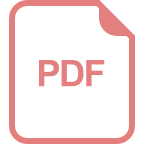
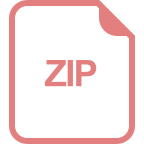
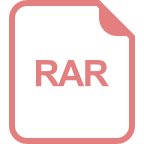
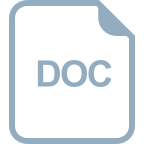
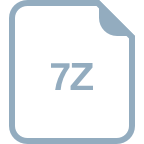
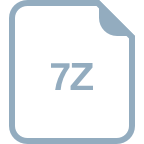