reference to type 'const QPixmap' could not bind to an lvalue of type 'QPixmap *'
时间: 2024-02-02 12:03:19 浏览: 57
抱歉,我之前提供的代码有误。您可以使用QLabel的setPixmap方法来插入图片,但是需要将QPixmap对象作为指针传递给setPixmap方法。
以下是修正后的示例代码:
```python
from PyQt5.QtWidgets import QApplication, QLabel
from PyQt5.QtGui import QPixmap
app = QApplication([])
label = QLabel()
pixmap = QPixmap("path/to/your/image.jpg") # 替换为您的图片路径
label.setPixmap(pixmap.scaled(label.size())) # 使用scaled方法将图片按比例缩放适应label大小
label.show()
app.exec()
```
修正后的代码将首先使用scaled方法将图像按比例缩放以适应label的大小,然后将其设置为QLabel的图像。
请确保将"path/to/your/image.jpg"替换为您实际的图片路径。此代码将创建一个QLabel并将图像显示在窗口中。
相关问题
上述代码怎么修改才能避免以下错误 error: non-const lvalue reference to type 'QPixmap' cannot bind to a temporary of type 'QPixmap'
这个错误是因为你尝试将一个临时对象(temporary object)绑定到一个非 const 的 lvalue 引用上,而 C++ 不允许这样做。
要避免这个错误,你可以将 QPixmap 对象声明为一个 const 引用,或者使用值传递的方式。例如,将代码修改为:
```
QPixmap newPixmap(this->width() + 1, this->width() + 1);
bg.swap(newPixmap);
```
这里我们先创建一个新的 QPixmap 对象 newPixmap,并将其大小设置为 (this->width() + 1, this->width() + 1)。然后通过 swap 函数将 bg 对象和 newPixmap 对象进行交换。这样就避免了使用临时对象导致的编译错误。
D:\aaagxzhsy\untitled\mainwindow.cpp:96: error: reference to type 'const QString' could not bind to an lvalue of type 'double'
这个错误提示表明,第96行代码中将一个 `double` 类型的值赋值给了一个 `const QString` 类型的变量或者函数参数,而这个 `const QString` 类型的变量或者函数参数是不能被修改的,因此无法将一个 `double` 类型的值直接赋值给它。
要解决这个问题,需要将 `double` 类型的值转换成 `QString` 类型的字符串,然后再将字符串赋值给 `const QString` 类型的变量或者函数参数。可以使用 `QString` 类的 `number()` 函数来将 `double` 类型的值转换成字符串,例如:
```c++
double value = 3.14;
const QString str = QString::number(value);
```
这样就可以将 `double` 类型的值 `3.14` 转换成字符串类型,并将其赋值给 `const QString` 类型的变量 `str`。
相关推荐
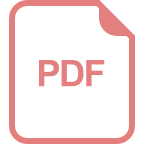
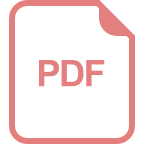
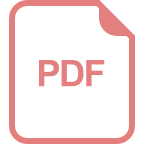













