5. 点名册一般用于登记人员的出勤情况,常见于大学课堂,大学老师通过点名册来统计出勤人数。点名册上的姓名通常会按照字母顺序排列。如果姓名全拼的首字母相同,会按照第2个字母排序。本任务要求读者编写一个点名册程序,样式如下所示。
时间: 2024-09-24 20:27:10 浏览: 43
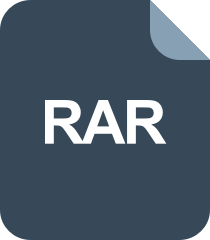
callName.rar_Java随机点名册_java点名系统_学生点名系统_点名 系统_点名系统

在创建一个简单的点名册程序时,我们可以设计一个结构来存储学生的姓名信息,包括姓名和出勤状态。这个程序的主要功能可以分为以下几个步骤:
1. **数据结构设计**:可以使用Python字典或者列表(如元组的列表),其中每个元素代表一个学生,包含姓名(字符串)和出勤状态(布尔值或整数表示出席、缺席等)。
```python
class Student:
def __init__(self, name):
self.name = name
self.attendance = None
# 或者使用列表存储
students = []
```
2. **添加学生**:允许用户输入新学生的姓名并将其添加到点名册。
```python
def add_student():
name = input("请输入学生姓名:")
students.append(Student(name))
```
3. **更新出勤状态**:允许查询并更新某个学生的出勤状况。
```python
def update_attendance(student_name):
for student in students:
if student.name == student_name:
# 更新出勤状态
new_status = input(f"{student_name} 的出勤状态是?(0/1) ")
student.attendance = int(new_status)
break
```
4. **查看出勤记录**:按字母顺序显示所有学生的姓名及出勤状态。
```python
def print_roll_call():
sorted_students = sorted(students, key=lambda s: (s.name[0], s.name[1:]))
for student in sorted_students:
print(f"{student.name}: {student.attendance}")
```
5. **主循环**:提供一个界面让教师进行操作,例如添加学生、查询状态或打印整个点名册。
```python
while True:
user_input = input("请选择操作:1. 添加学生;2. 更新出勤;3. 查看记录;4. 退出\n")
if user_input == "1":
add_student()
elif user_input == "2":
name = input("请输入学生姓名:")
update_attendance(name)
elif user_input == "3":
print_roll_call()
elif user_input == "4":
break
else:
print("无效输入,请重新选择。")
```
阅读全文
相关推荐
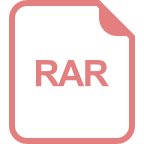
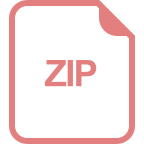
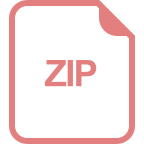
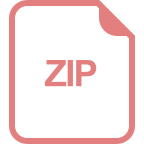
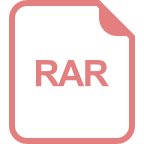
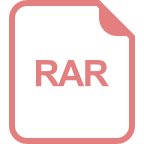
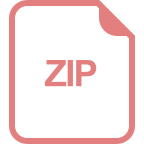
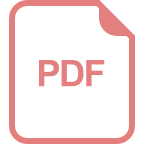
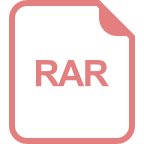
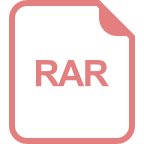
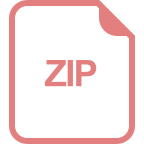
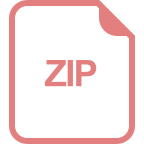
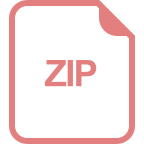
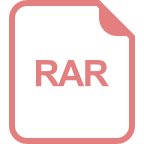
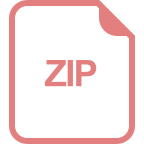
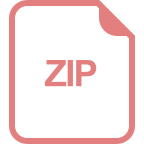