我想写一个C++代码,从字符串中提取出数字,包括16进制的数字(以0x开头)std::string input = “Here are some numbers: 123, 0x1A3, 4567, and 0xFF.”
时间: 2024-09-09 11:13:30 浏览: 83
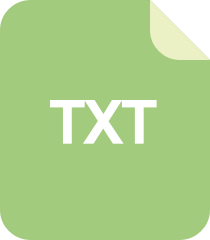
统计字符串中字母和数字个数
你可以使用C++标准库中的`std::stringstream`和`std::regex`来实现这个功能。下面是一个简单的示例:
```cpp
#include <iostream>
#include <sstream>
#include <regex>
std::string input = "Here are some numbers: 123, 0x1A3, 4567, and 0xFF.";
// 正则表达式匹配数字
std::regex digit_pattern("(\\d+)|(0x[0-9A-Fa-f]+)");
void extract_numbers(const std::string& str) {
std::smatch matches;
std::stringstream ss(str);
std::string match;
// 使用正则匹配每个数字并保存到match中
while (std::regex_search(ss, matches, digit_pattern)) {
if (matches.size() > 1 && matches[2].length()) { // 检查是否是十六进制
match = "0x" + matches[2]; // 添加前缀0x
} else {
match = matches[0];
}
std::cout << match << std::endl;
}
}
int main() {
extract_numbers(input);
return 0;
}
```
这段代码首先定义了一个正则表达式模式,它可以匹配十进制整数和十六进制数。然后在一个循环中,它遍历输入字符串并尝试找出所有匹配项。如果是十六进制数,会在前面添加`0x`。每一个找到的数字会被打印出来。
阅读全文
相关推荐
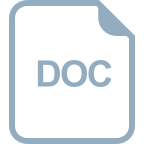
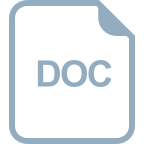















