springboot 通用脚手架
时间: 2023-11-19 16:57:22 浏览: 98
以下是一个基于Spring Boot的通用脚手架的示例:
1. 首先,你需要在pom.xml文件中添加以下依赖项:
```xml
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.4.2</version>
</dependency>
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.3.0</version>
</dependency>
```
2. 接下来,你需要创建一个实体类,例如:
```java
public class User {
private Long id;
private String name;
private Integer age;
// 省略getter和setter方法
}
```
3. 然后,你需要创建一个Mapper接口,例如:
```java
@Mapper
public interface UserMapper extends BaseMapper<User> {
}
```
4. 接下来,你需要创建一个Service接口和实现类,例如:
```java
public interface UserService extends IService<User> {
}
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService {
}
```
5. 最后,你需要创建一个Controller类,例如:
```java
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("")
public List<User> list() {
return userService.list();
}
@PostMapping("")
public boolean save(@RequestBody User user) {
return userService.save(user);
}
@GetMapping("/{id}")
public User get(@PathVariable Long id) {
return userService.getById(id);
}
@PutMapping("/{id}")
public boolean update(@PathVariable Long id, @RequestBody User user) {
user.setId(id);
return userService.updateById(user);
}
@DeleteMapping("/{id}")
public boolean delete(@PathVariable Long id) {
return userService.removeById(id);
}
}
```
这个示例演示了如何使用Spring Boot和MyBatis Plus快速创建一个通用脚手架,它可以处理基本的CRUD操作。
阅读全文
相关推荐
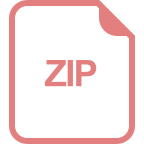
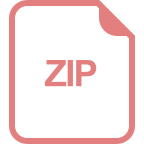
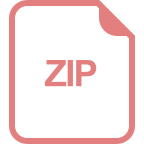
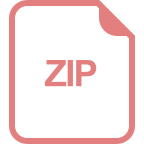
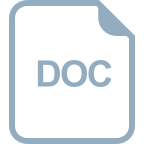
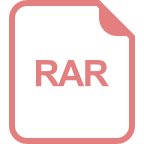
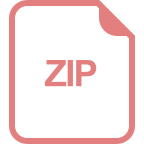
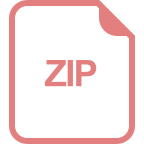
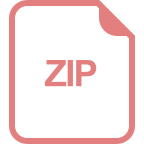
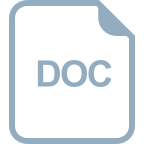
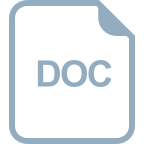
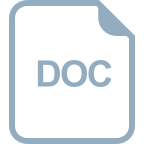
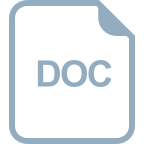
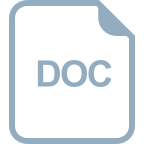
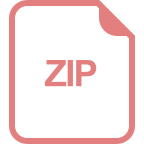
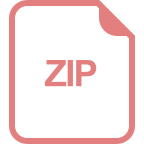
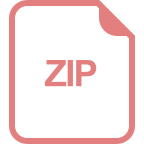