在C语言编程中,如何实现一个循环队列的数据结构?包括从用户键盘输入元素并进行添加(enqueue)操作,以及删除(dequeue)队首元素的过程,请提供详细的代码示例和步骤说明。
时间: 2024-10-25 11:15:17 浏览: 40
在C语言中,我们可以使用数组和两个指针(一个指向队首,另一个指向队尾)来实现循环队列。由于数组下标是有限的,所以我们需要设置一个哨兵元素来处理队列满和空的情况。以下是实现一个循环队列的步骤及代码示例:
1. 定义结构体,包含数据域和两个指针,队头和队尾。
```c
typedef struct {
int *items; // 存储元素的数组
int front; // 队头指针
int rear; // 队尾指针
int capacity; // 队列容量
} Queue;
```
2. 初始化队列:创建一个新的队列,分配内存给存储元素的数组,并设置队头和队尾指针。
```c
Queue* createQueue(int size) {
Queue *q = malloc(sizeof(Queue));
q->items = malloc(size * sizeof(int));
q->front = -1;
q->rear = -1;
q->capacity = size;
return q;
}
```
3. 向队列添加元素(enqueue):检查队列是否已满,如果未满则移动队尾指针,否则插入新元素后更新队尾指针。
```c
void enqueue(Queue *q, int item) {
if ((q->rear + 1) % q->capacity == q->front) {
printf("队列已满,无法添加元素.\n");
return;
}
q->rear = (q->rear + 1) % q->capacity; // 循环队列处理
q->items[q->rear] = item;
}
```
4. 从队列删除元素(dequeue):检查队列是否为空,如果非空则移动队头指针,返回并移除第一个元素,否则输出错误信息。
```c
int dequeue(Queue *q) {
if (q->front == -1) {
printf("队列为空,无法删除元素.\n");
return -1;
}
int removedItem = q->items[q->front];
q->front = (q->front + 1) % q->capacity; // 循环队列处理
return removedItem;
}
```
5. 用户交互部分:从键盘获取输入并进行添加和删除操作。
```c
int main() {
Queue *queue = createQueue(5);
while (1) {
printf("选择操作:\n1. 添加元素\n2. 删除元素\n3. 查看队列\n4. 退出\n");
int choice;
scanf("%d", &choice);
switch (choice) {
case 1: {
int item;
printf("请输入元素: ");
scanf("%d", &item);
enqueue(queue, item);
break;
}
case 2: {
int result = dequeue(queue);
if (result != -1)
printf("移除的元素: %d\n", result);
else
printf("未找到元素.\n");
break;
}
// 其他case...
}
}
free(queue->items);
free(queue);
return 0;
}
```
阅读全文
相关推荐
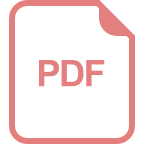
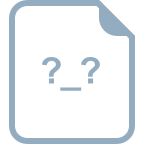
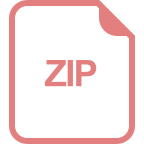
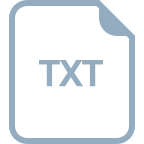
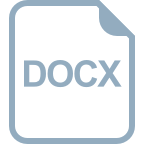
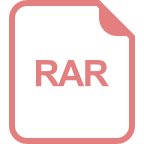
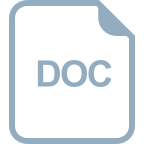
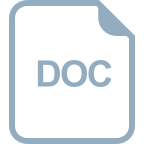
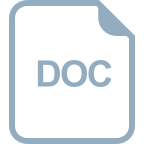
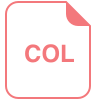
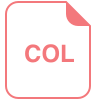

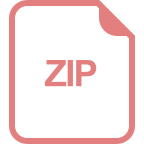
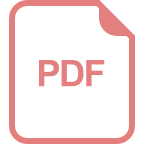
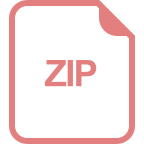
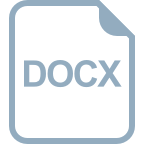