C# 实现SCP下载文件到本地
时间: 2024-09-10 11:06:17 浏览: 76
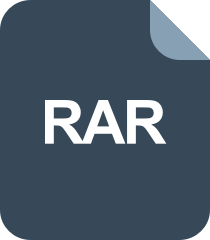
C# SCP文件传输,包括下载和上传
在C#中,要实现Secure Copy (SCP) 协议下载文件到本地,你可以使用第三方库如`SCPNet`。首先,你需要安装这个库,可以通过NuGet包管理器添加:
```powershell
Install-Package SCPNet
```
然后,你可以创建一个简单的示例来下载文件,这里是一个基本的步骤:
1. 引入所需的命名空间:
```csharp
using SCPNet;
using SCPNet.Authentication;
using System;
using System.IO;
```
2. 创建SCP客户端并设置认证信息(例如SSH密钥对或用户名密码):
```csharp
var client = new ScpClient("scp.example.com", 22); // 服务器地址和端口
var privateKey = File.ReadAllText(@"path/to/private.key"); // 私钥路径
// 使用私钥认证
client.Connect(privateKey);
```
3. 调用Download方法下载文件:
```csharp
string remoteFilePath = "/path/to/remote/file.txt";
string localDestinationPath = @"C:\local\file.txt"; // 本地保存位置
if (!client.Exists(remoteFilePath)) {
Console.WriteLine($"File {remoteFilePath} not found on the server.");
} else {
using var stream = client.Download(remoteFilePath);
if (stream != null) {
using var fileStream = File.Create(localDestinationPath);
stream.CopyTo(fileStream);
Console.WriteLine($"File downloaded successfully from {remoteFilePath} to {localDestinationPath}");
} else {
Console.WriteLine("Error occurred while downloading file.");
}
}
```
4. 下载完成后关闭连接:
```csharp
client.Disconnect();
```
阅读全文
相关推荐
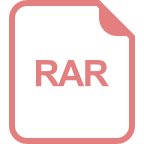
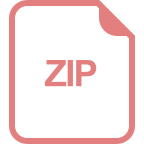
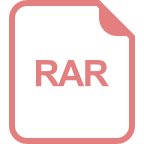
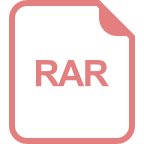
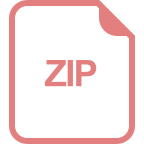
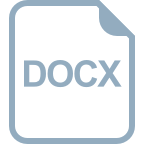
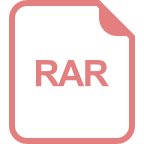
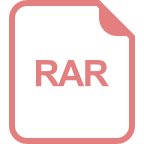
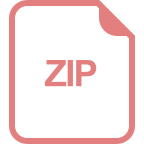
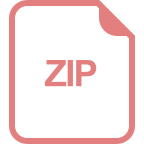
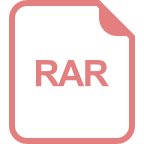
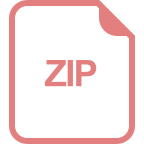
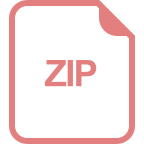
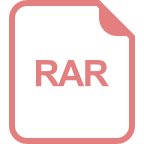
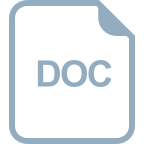
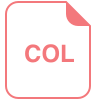

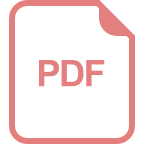