c++使用std::find_if查找结构体数组中首个结构体中整型为0的结构体在数组中的索引
时间: 2024-11-27 22:13:45 浏览: 30
在 C++ 中,你可以使用 `std::find_if` 函数配合范围-based for 循环来查找结构体数组中第一个整型字段为0的结构体的位置。`std::find_if` 是 `<algorithm>` 库中的函数,它接受一个范围(如数组或容器)以及一个谓词(即一个返回 bool 的函数对象),用于遍历并检查每个元素是否满足条件。
首先,假设你有一个名为 `StructType` 的结构体,其中包含一个整型字段 `intValue`:
```cpp
struct StructType {
int intValue;
};
```
然后,你可以创建一个 `StructType` 类型的数组,并找到第一个 `intValue` 为 0 的元素的索引:
```cpp
std::vector<StructType> array; // 假设已填充了数据
auto it = std::find_if(array.begin(), array.end(),
[](const StructType& s) { return s.intValue == 0; });
if (it != array.end()) {
size_t index = std::distance(array.begin(), it); // 索引从0开始计数
std::cout << "Found at index: " << index << '\n';
} else {
std::cout << "No element found with zero intValue.\n";
}
```
这里的关键在于 `[](const StructType& s) { return s.intValue == 0; }` 这是一个lambda表达式,作为 `std::find_if` 的谓词。
阅读全文
相关推荐
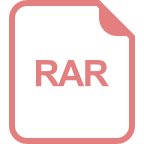
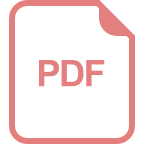
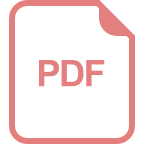
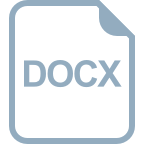
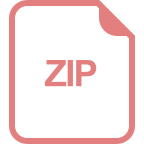
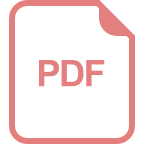
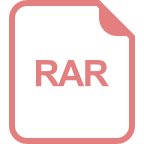
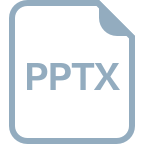
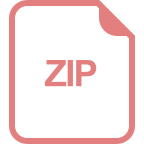
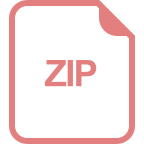
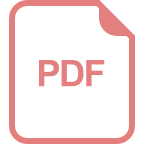
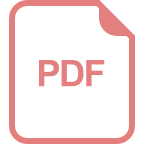
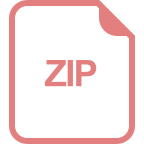
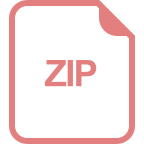
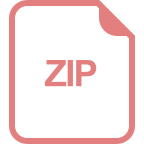