编写程序,输入一个学生的语文,数学,英语,物理成绩,计算并输出该学生的总成绩和平均成绩。
时间: 2024-10-15 15:13:05 浏览: 23
编写一个简单的Python程序可以这样实现:
```python
def calculate_student_score():
# 输入学生各科成绩
chinese = float(input("请输入学生的语文成绩:"))
math = float(input("请输入学生的数学成绩:"))
english = float(input("请输入学生的英语成绩:"))
physics = float(input("请输入学生的物理成绩:"))
# 计算总成绩
total_score = chinese + math + english + physics
# 计算平均成绩(四门课程)
average_score = total_score / 4
print(f"该学生的总成绩是:{total_score}")
print(f"该学生的平均成绩是:{average_score}")
calculate_student_score()
```
在这个程序中,我们首先通过`input()`函数获取用户输入的学生各科成绩,并转换成浮点数以便进行加法运算。然后计算总成绩和平均成绩,并使用`print()`函数输出结果。
相关问题
编写C语言程序,输入一个学生的语文、数学、英语、物理成绩,计算并输出该学生的总成绩和平均成绩
```c
#include <stdio.h>
int main()
{
float chinese, math, english, physics, total, average;
printf("请输入学生的语文、数学、英语、物理成绩:\n");
scanf("%f%f%f%f", &chinese, &math, &english, &physics);
total = chinese + math + english + physics;
average = total / 4;
printf("该学生的总成绩为:%.2f\n", total);
printf("该学生的平均成绩为:%.2f\n", average);
return 0;
}
```
说明:
1. 定义变量 `chinese`、`math`、`english`、`physics` 分别表示学生的语文、数学、英语、物理成绩。
2. 使用 `printf` 函数提示用户输入学生的成绩,使用 `scanf` 函数读取用户输入的成绩。
3. 计算学生的总成绩和平均成绩,并将结果分别存储在变量 `total` 和 `average` 中。
4. 使用 `printf` 函数输出学生的总成绩和平均成绩。其中 `"%.2f"` 表示输出浮点数,保留两位小数。
用c++编写程序,输入一个学生的语文,数学,英语,物理成绩,计算并输出该学生的总成绩和平均成绩。
在C++中,你可以使用结构体定义一个学生对象,并通过cin从用户那里获取分数,然后计算总分和平均分。这里是一个简单的示例:
```cpp
#include <iostream>
using namespace std;
// 定义一个结构体表示学生
struct Student {
float chinese;
float math;
float english;
float physics;
};
void calculateScore(Student student) {
// 计算总分
float total_score = student.chinese + student.math + student.english + student.physics;
// 计算平均分,注意除数需要加1防止分母为0的情况
float average_score = (total_score / 4.0) + 0.0001; // 为了避免浮点数除法的精度误差
cout << "学生的总成绩是: " << total_score << endl;
cout << "学生的平均成绩是: " << average_score << endl;
}
int main() {
// 创建Student对象并获取输入
Student stu;
cout << "请输入学生的语文成绩: ";
cin >> stu.chinese;
cout << "请输入学生的数学成绩: ";
cin >> stu.math;
cout << "请输入学生的英语成绩: ";
cin >> stu.english;
cout << "请输入学生的物理成绩: ";
cin >> stu.physics;
// 调用函数计算并输出结果
calculateScore(stu);
return 0;
}
```
阅读全文
相关推荐
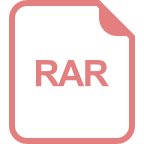
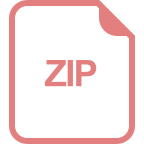
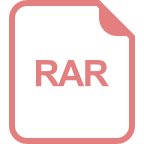








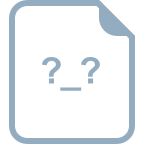
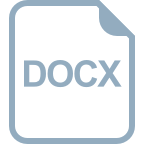
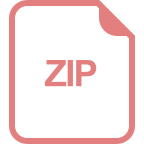



